java参数 List<Student>, int a功能: 将指定a索引位置的学生删除 返回删除成功 或者 删除失败
时间: 2023-05-22 20:05:18 浏览: 100
这个功能可以通过以下代码实现:
public static boolean removeStudent(List<Student> list, int index) {
if (index < 0 || index >= list.size()) {
return false; // 删除失败,索引越界
}
list.remove(index);
return true; // 删除成功
}
其中,List<Student>参数表示学生列表,int参数a表示要删除的学生的索引位置。如果索引越界或者删除失败,返回false,否则返回true。
相关问题
练习-Java集合类之List的ArrayList之删除指定学生
在Java中,如果要在`ArrayList<Student>`列表中删除指定的学生,你可以使用`remove(Object)`方法。这个方法会从列表中移除第一个匹配给定对象的元素。这里假设有一个名为`Student`的类,它有一个表示姓名的属性。
```java
import java.util.ArrayList;
class Student {
private String name;
// 构造函数和getter/setter省略
@Override
public boolean equals(Object obj) {
if (obj instanceof Student) {
return this.name.equals(((Student) obj).getName());
}
return false;
}
@Override
public int hashCode() {
return name.hashCode();
}
}
public class Main {
public static void main(String[] args) {
// 创建ArrayList并填充数据
ArrayList<Student> students = new ArrayList<>();
students.add(new Student("张三"));
students.add(new Student("李四"));
students.add(new Student("王五"));
// 删除指定学生,这里假设我们要删除"王五"
String targetName = "王五";
for (int i = 0; i < students.size(); i++) {
if (students.get(i).equals(new Student(targetName))) {
students.remove(i); // 使用索引i删除对应位置的学生
break; // 找到目标后立即停止搜索
}
}
// 输出剩余的学生
for (Student student : students) {
System.out.println(student.getName());
}
}
}
```
在这个例子中,我们首先创建了一个`ArrayList<Student>`,然后通过遍历查找目标学生的名称,并调用`remove()`方法将其从列表中移除。需要注意的是,由于列表可能会被改变大小,所以在删除元素之后需要更新循环的条件,防止访问到已经被移除的位置。
c++请帮助张老师把这些学生信息储存到一个string类型的向量 list 中,并输出学号序号为3和4的学生的名字 输出样例: Student A Student B
在C++中,你可以创建一个自定义的`Student`类来存储学生的姓名,然后将这些信息存储在一个`std::vector<std::string>`类型的list中。这里假设每个学生的信息是一个字符串,例如"Student Name"。首先,你需要定义`Student`类,然后编写函数来添加学生并提取特定学生的姓名。
```cpp
#include <iostream>
#include <vector>
#include <string>
// 定义Student类
class Student {
public:
std::string name;
int id;
// 构造函数
Student(int id, const std::string& name) : id(id), name(name) {}
};
// 函数来存储学生信息
void storeStudents(std::vector<std::string>& studentList, const std::vector<Student>& students) {
for (const auto& student : students) {
studentList.push_back("Student " + std::to_string(student.id) + " " + student.name);
}
}
// 函数来输出指定学号的学生名字
void printStudents(const std::vector<std::string>& studentList, int index1, int index2) {
if (index1 >= 0 && index1 < studentList.size() && index2 >= 0 && index2 < studentList.size()) {
std::cout << "Student " << index1 << ": " << studentList[index1] << std::endl;
std::cout << "Student " << index2 << ": " << studentList[index2] << std::endl;
} else {
std::cout << "Invalid index!" << std::endl;
}
}
int main() {
// 创建学生数据
std::vector<Student> students = {Student(1, "A"), Student(2, "B"), Student(3, "C"), Student(4, "D")};
// 存储学生信息到字符串列表
std::vector<std::string> studentNames;
storeStudents(studentNames, students);
// 输出第3和第4名学生的名字
printStudents(studentNames, 2, 3); // 注意索引是从0开始的
return 0;
}
```
在这个例子中,`storeStudents`函数用于添加学生信息,而`printStudents`函数则根据给定的学号索引打印对应的学生名字。注意在`printStudents`函数里,由于数组下标从0开始,所以输出学号序列为3和4的学生,我们需要传入索引值2和3。
阅读全文
相关推荐
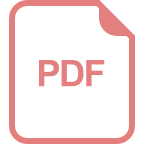
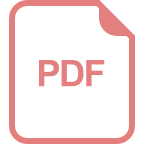
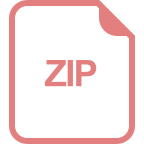













