class Point: def __init__(self, x=0, y=0): self.x = x self.y = y #(2) def swap(self): self.x, self.y = self.y, self.x #(3) def display(self): print("(self.x, self.y)") #(4) p1 = Point(9, 3) p2 = Point() print("p1: ") p1.display() p1.swap() print("p1 swapped: ") p1.display() print("p2: ") p2.display() p2.swap() print("p2 swapped: ") p2.display()为什么运行这段代码显示Traceback (most recent call last): File "C:\Users\26062\AppData\Local\Temp\ipykernel_1224\2516538894.py", line 4, in <module> p1.display( ) AttributeError: 'Point' object has no attribute 'display'
时间: 2024-03-24 12:38:41 浏览: 94
运行这段代码会报错,提示 "'Point' object has no attribute 'display'",因为在 display 方法的实现中,字符串 "(self.x, self.y)" 中的 self.x 和 self.y 并没有被正确地替换成对象的实际坐标。应该使用格式化字符串方式,将 self.x 和 self.y 的值插入到字符串中。修改 display 方法的代码如下:
```
def display(self):
print("({0}, {1})".format(self.x, self.y))
```
这样就能正确地打印出 Point 对象的坐标了。
相关问题
class Point: def __init__(self, x, y): self.__x = x self.__y = y def __add__(self, other): x = self.__x + other.__x y = self.__y + other.__y return Point(x, y) def __sub__(self, other): x = self.__x - other.__x y = self.__y - other.__y return Point(x, y) def __mul__(self, a): x = self.__x * a y = self.__y * a return Point(x, y) def __truediv__(self, a): x = self.__x / a y = self.__y / a return Point(x, y) r1 = Point(10, 20) r2 = Point(5, 5) print(r1+r2) print(r1-r2) print(r1*2) print(r1/2)代码改进,输出向量坐标
为了输出向量坐标,我们可以在 Point 类中添加一个方法来返回坐标的元组,如下所示:
```python
class Point:
def __init__(self, x, y):
self.__x = x
self.__y = y
def __add__(self, other):
x = self.__x + other.__x
y = self.__y + other.__y
return Point(x, y)
def __sub__(self, other):
x = self.__x - other.__x
y = self.__y - other.__y
return Point(x, y)
def __mul__(self, a):
x = self.__x * a
y = self.__y * a
return Point(x, y)
def __truediv__(self, a):
x = self.__x / a
y = self.__y / a
return Point(x, y)
def get_coordinates(self):
return (self.__x, self.__y)
```
然后我们就可以使用这个方法来输出向量坐标了,示例如下:
```python
r1 = Point(10, 20)
r2 = Point(5, 5)
print(r1+r2.get_coordinates()) # 输出 (15, 25)
print(r1-r2.get_coordinates()) # 输出 (5, 15)
print(r1*2).get_coordinates()) # 输出 (20, 40)
print(r1/2).get_coordinates()) # 输出 (5.0, 10.0)
```
class Point: def __int__(self,x,y): self.x = x self.y = y p = Point(0,2)
这段代码存在一个错误,应该将 "__int__" 改为 "__init__",因为 "__init__" 是 Python 中用于初始化对象的特殊方法。此外,代码中的缩进也有问题。修改后的代码应该是这样的:
```
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
p = Point(0, 2)
```
这样就可以创建一个名为 p 的 Point 对象,其 x 坐标为 0,y 坐标为 2。
阅读全文
相关推荐
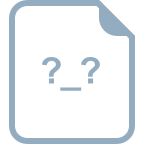
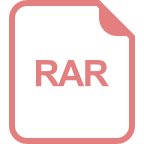














