pd.read_excel指定两列读取
时间: 2023-12-17 09:03:36 浏览: 71
Sure, I can help you with that! To read only specific columns from an Excel file using pd.read_excel, you need to use the parameter "usecols" and pass in a list of the column names or indices that you want to read. For example, if your Excel file has columns named "Name", "Age", "Gender", and "Salary", and you only want to read the "Name" and "Salary" columns, you can use the following code:
```
import pandas as pd
df = pd.read_excel('your_file.xlsx', usecols=['Name', 'Salary'])
```
Hope this helps! Let me know if you have any more questions.
相关问题
import pandas as pd df = pd.read_excel(r"C:\Users\asus\Desktop\用户账号情况统计表.xlsx") # 选择所属组织列和用户列 data = df[['用户账号', '姓名']] # 将内容转换为字典 organization_user_dict = data.set_index('用户账号')['姓名'].to_dict() print(organization_user_dict) df2 = pd.read_excel(r"C:\Users\asus\Desktop\人员分配企业岗位查询表.xlsx") print(df2)
这段代码使用 Pandas 库读取了两个 Excel 文件,并将第一个文件中的“用户账号”和“姓名”这两列内容转换为字典,其中“用户账号”列作为字典的键(key),“姓名”列作为字典的值(value)。然后,打印了转换后的字典内容。
接下来,又读取了第二个 Excel 文件,并将其内容保存在名为 `df2` 的 DataFrame 中,然后直接打印了 `df2` 的内容。
需要注意的是,这段代码中的 Excel 文件路径需要根据实际情况进行修改,确保能够正确读取到文件。
pd.read_excel(converters)
`pd.read_excel(converters)` 是 pandas 库中用于读取 Excel 文件的方法。`converters` 是一个参数,用于指定在读取 Excel 文件时对特定列应用的转换函数。
转换函数可以是一个字典,其中键是列名,值是应用于该列的转换函数。转换函数可以是任何可调用对象,如函数、lambda 函数或自定义类的方法。它们用于在读取 Excel 文件时对特定列的值进行转换或处理。
以下是一个示例:
```python
import pandas as pd
# 定义一个转换函数
def convert_func(value):
# 假设需要将字符串转换为大写
return str(value).upper()
# 读取 Excel 文件,并在读取过程中应用转换函数
data = pd.read_excel('file.xlsx', converters={'Column1': convert_func, 'Column2': lambda x: x*2})
```
上述示例中,`converters` 参数指定了两列的转换函数。'Column1' 列使用了名为 `convert_func` 的自定义转换函数,而 'Column2' 列使用了一个 lambda 函数,将其值乘以 2。读取 Excel 文件后,`data` 变量将包含经过转换的数据。
阅读全文
相关推荐














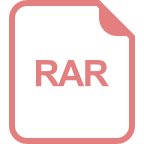