按照RRT*算法中的优化重连算法优化这行代码:%step7.重连接,以Pnew为父节点 for i = i:size(T.v,2)-1 dist = sqrt((Pnew(1) - T.v(i).x)^2 + (Pnew(2) - T.v(i).y)^2); if dist < r && i ~= minInd %计算当前点经过Pnew的代价,如果更小就更新 this_cost = dist + tmp_cost; if this_cost < T.v(i).cost %如果没有发生碰撞 this_p = [T.v(i).x,T.v(i).y]; if iscollision2(this_p,Pnew,dist,Img) continue; end T.v(i).cost = this_cost; T.v(i).xPre = Pnew(1); T.v(i).yPre = Pnew(2); T.v(i).indPre = k; end end end
时间: 2024-04-27 22:22:28 浏览: 12
这段代码是RRT*算法中的优化重连算法,在重连时以Pnew为父节点,遍历树中除了Pnew的所有节点,计算当前节点到Pnew的距离dist,如果小于阈值r且不是Pnew本身,则计算当前点经过Pnew的代价this_cost,并与当前点的代价比较,如果更小就更新当前点的代价、父节点和路径。如果更新后没有发生碰撞,则将当前点的代价、父节点和路径更新为Pnew的代价、父节点和路径。
为了优化这段代码,可以考虑以下几点:
1. 使用 KD 树或 R 树等数据结构来加速树的遍历和搜索,减少重连的计算量。
2. 对于已经计算过的节点,可以将它们的代价存储下来,避免重复计算。
3. 可以考虑引入一些启发式方法,如 A* 算法,来优化路径搜索过程。
4. 可以在代价计算时加入一些额外的约束条件,如车辆转向、速度等,来更加准确地计算路径代价。
通过以上优化措施,可以加速路径搜索过程,提高算法的效率和准确性。
相关问题
RRT*算法程序代码
RRT*(Rapidly-exploring Random Tree Star)算是一种用于路径规划的算法,它在RRT算法的基上进行了改进,能够更好地优化路径。下面是一个简单的RRT*算法的程序代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
class Node:
def __init__(self, x, y):
self.x = x
self.y = y
self.parent = None
class RRTStar:
def __init__(self, start, goal, obstacles, max_iter=1000, step_size=0.5, goal_sample_rate=0.05, radius=2.0):
self.start = Node(start[0], start[1])
self.goal = Node(goal[0], goal[1])
self.obstacles = obstacles
self.max_iter = max_iter
self.step_size = step_size
self.goal_sample_rate = goal_sample_rate
self.radius = radius
self.nodes = []
def planning(self):
self.nodes.append(self.start)
for i in range(self.max_iter):
rnd_node = self.generate_random_node()
nearest_node = self.find_nearest_node(rnd_node)
new_node = self.steer(nearest_node, rnd_node)
if self.check_collision(new_node):
near_nodes = self.find_near_nodes(new_node)
min_cost_node = nearest_node
min_cost = self.calculate_cost(nearest_node) + self.calculate_distance(nearest_node, new_node)
for node in near_nodes:
cost = self.calculate_cost(node) + self.calculate_distance(node, new_node)
if cost < min_cost:
min_cost_node = node
min_cost = cost
new_node = self.steer(min_cost_node, new_node)
self.nodes.append(new_node)
self.rewire(new_node, near_nodes)
if self.calculate_distance(new_node, self.goal) <= self.step_size:
final_node = self.steer(new_node, self.goal)
if self.check_collision(final_node):
self.nodes.append(final_node)
return self.generate_path()
return None
def generate_random_node(self):
if np.random.rand() < self.goal_sample_rate:
return Node(self.goal.x, self.goal.y)
else:
x = np.random.uniform(0, 10)
y = np.random.uniform(0, 10)
return Node(x, y)
def find_nearest_node(self, rnd_node):
distances = [self.calculate_distance(node, rnd_node) for node in self.nodes]
nearest_node = self.nodes[np.argmin(distances)]
return nearest_node
def steer(self, from_node, to_node):
distance = self.calculate_distance(from_node, to_node)
if distance <= self.step_size:
return to_node
else:
theta = np.arctan2(to_node.y - from_node.y, to_node.x - from_node.x)
x = from_node.x + self.step_size * np.cos(theta)
y = from_node.y + self.step_size * np.sin(theta)
return Node(x, y)
def check_collision(self, node):
for obstacle in self.obstacles:
if self.calculate_distance(node, obstacle) <= self.radius:
return False
return True
def find_near_nodes(self, node):
distances = [self.calculate_distance(node, n) for n in self.nodes]
near_nodes = [n for n, d in zip(self.nodes, distances) if d <= 2 * self.radius]
return near_nodes
def calculate_cost(self, node):
cost = 0
while node.parent:
cost += self.calculate_distance(node, node.parent)
node = node.parent
return cost
def rewire(self, new_node, near_nodes):
for node in near_nodes:
cost = self.calculate_cost(new_node) + self.calculate_distance(new_node, node)
if cost < self.calculate_cost(node):
node.parent = new_node
def calculate_distance(self, node1, node2):
return np.sqrt((node1.x - node2.x) ** 2 + (node1.y - node2.y) ** 2)
def generate_path(self):
path = []
node = self.nodes[-1]
while node:
path.append([node.x, node.y])
node = node.parent
path.reverse()
return path
# 示例使用
start = (1, 1)
goal = (9, 9)
obstacles = [(5, 5), (7, 7)]
rrt_star = RRTStar(start, goal, obstacles)
path = rrt_star.planning()
if path is not None:
print("找到路径!")
for point in path:
print(point)
else:
print("未找到路径!")
```
这段代码实现了一个简单的RRT*算法,其中包括节点类`Node`和RRT*类`RRTStar`。在示例中,我们定义了起点、终点和障碍物,并通过调用`planning`方法来进行路径规划。如果找到了路径,将输出路径上的点坐标;否则,将输出未找到路径的提示。
优化Quick-RRT*算法c++
Quick-RRT*是一种改进版的Rapidly-exploring Random Trees (RRT)算法,它采用了更高效的近似最优路径搜索方法。以下是一些优化Quick-RRT*算法的建议:
1. 优化距离度量函数: Quick-RRT*使用的是欧几里得距离度量函数,但这在高维空间中不够有效。你可以尝试使用其他距离度量函数,比如L1或L∞度量函数。
2. 优化采样策略: Quick-RRT*使用的是均匀采样策略,但这在空间中的障碍物密集区域和窄通道中可能会导致采样效率低下。你可以尝试使用更智能的采样策略,比如基于机器学习的采样策略或基于先验知识的采样策略。
3. 优化路径选择策略: Quick-RRT*使用的是最短路径选择策略,但这可能会导致路径相对较长。你可以尝试使用其他路径选择策略,比如最小路径选择策略或基于在线规划的路径选择策略。
4. 优化优化器: Quick-RRT*采用了类似于梯度下降的优化器来搜索最优路径,但这可能会陷入局部最优解。你可以尝试使用其他优化器,比如遗传算法或模拟退火算法。
5. 优化数据结构: Quick-RRT*的性能高度依赖于数据结构的性能。你可以尝试使用更高效的数据结构,比如KD树或R树,来加速路径搜索。
这些优化策略可以单独或同时使用,以提高Quick-RRT*算法的性能。
相关推荐
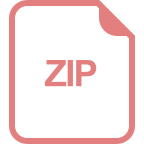
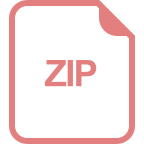












