x = torch.cat((x, grid), dim=-1)
时间: 2023-11-26 14:04:17 浏览: 30
这行代码使用 PyTorch 的 `cat` 函数将 `x` 和 `grid` 沿着最后一个维度进行拼接。具体来说,如果 `x` 的形状是 `(batch_size, channel_in, height, width)`,`grid` 的形状是 `(batch_size, num_points, 2)`,那么拼接后的形状就是 `(batch_size, channel_in+2, height, width)`。
这个操作常用于将图像特征和空间位置信息合并到一起,比如在实现空间注意力机制时会用到。
相关问题
grid = self.get_grid(x.shape, x.device) x = torch.cat((x, grid), dim=-1) x = self.p(x) x = x.permute(0, 3, 1, 2)
这段代码的作用是什么?
这段代码的作用是在输入张量 x 的最后一个维度上拼接一个网格矩阵,然后将结果输入到一个卷积神经网络中。具体来说:
- self.get_grid(x.shape, x.device) 返回一个与 x 的形状相同的网格矩阵,其中每个元素的值表示该元素在张量中的坐标;
- torch.cat((x, grid), dim=-1) 在最后一个维度上将 x 和网格矩阵 grid 进行拼接;
- self.p(x) 对拼接后的张量进行卷积操作;
- x.permute(0, 3, 1, 2) 将通道维移动到第二个维度上,以符合 PyTorch 的张量表示规范。
这段代码可能用于图像分割模型中,将输入图像的每个像素点的坐标信息以及其他特征信息一起输入到卷积神经网络中进行处理,以提高模型的精度。
def decode_outputs(self, outputs, dtype): grids = [] strides = [] for (hsize, wsize), stride in zip(self.hw, self.strides): yv, xv = torch.meshgrid([torch.arange(hsize, dtype=dtype), torch.arange(wsize, dtype=dtype)]) grid = torch.stack((xv, yv), dim=2).view(1, -1, 2) grids.append(grid) shape = grid.shape[:2] strides.append(torch.full((*shape, 1), stride, dtype=dtype)) grids = torch.cat(grids, dim=1) strides = torch.cat(strides, dim=1) outputs[..., :2].add_(grids).mul_(strides) outputs[..., 2:4].exp_().mul_(strides) return outputs通过张量列表的形式替换for循环速度优化并提供代码
def decode_outputs(self, outputs, dtype):
hw = self.hw
strides = self.strides
grids = [torch.stack((torch.meshgrid([torch.arange(hsize, dtype=dtype), torch.arange(wsize, dtype=dtype)])), dim=2).view(1, -1, 2) for (hsize, wsize) in hw]
grids = torch.cat(grids, dim=1)
strides = torch.cat([torch.full((*grid.shape[:2], 1), stride, dtype=dtype) for stride, grid in zip(strides, grids)], dim=1)
outputs[..., :2] = (outputs[..., :2] + grids) * strides
outputs[..., 2:4] = torch.exp(outputs[..., 2:4]) * strides
return outputs
相关推荐
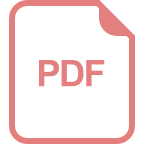
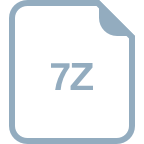












