由括号表达式递归创建二叉树并显示
时间: 2023-11-12 20:03:52 浏览: 84
可以使用递归算法来创建二叉树,具体步骤如下:
1. 从左往右扫描括号表达式,读取一个字符。
2. 如果字符是左括号,说明该节点是某个节点的左子节点,创建一个新节点,并递归地调用该函数来设置该节点的左子树。
3. 如果字符是右括号,说明该节点的左子树已经创建完了,返回上一层递归。
4. 如果字符是数字或负号,说明该节点是叶子节点,创建一个新节点,并将该字符作为该节点的值。
5. 如果字符是空格或其他无效字符,直接跳过。
6. 如果括号表达式已经扫描完毕,返回根节点。
代码示例如下:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def create_tree(s):
stack = []
i = 0
while i < len(s):
c = s[i]
if c == '(':
node = TreeNode()
if stack and stack[-1].left is None:
stack[-1].left = node
elif stack and stack[-1].right is None:
stack[-1].right = node
stack.append(node)
elif c == ')':
stack.pop()
elif c.isdigit() or c == '-':
j = i
while j < len(s) and (s[j].isdigit() or s[j] == '-'):
j += 1
num = int(s[i:j])
node = TreeNode(num)
if stack and stack[-1].left is None:
stack[-1].left = node
elif stack and stack[-1].right is None:
stack[-1].right = node
i += 1
return stack[0]
def inorder_traversal(root):
if root:
inorder_traversal(root.left)
print(root.val, end=' ')
inorder_traversal(root.right)
s = "(3(4(-5)(-6))(2(7)(8)))"
root = create_tree(s)
inorder_traversal(root) # 输出: -5 4 -6 3 7 2 8
```
相关推荐
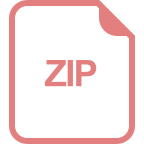
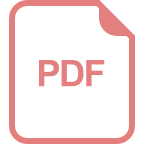
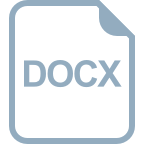














