针对按行分好特征词的txt文档编写一个贝叶斯分类模型完成对文本的分类并写出代码
时间: 2024-03-29 09:40:18 浏览: 140
好的,针对这个问题,我可以给您提供一份基于Python的贝叶斯分类模型代码,可以用于按行分好特征词的txt文档进行分类。
```python
import os
import math
class NaiveBayes:
def __init__(self):
self.vocab = set() # 词汇表
self.word_count = {} # 每个分类中的单词数
self.class_count = {} # 每个分类中的文档数
# 将文本拆分为单词列表
def text_parse(self, text):
words = text.split('\n')
return [word for word in words if len(word) > 0]
# 计算单词在文档中出现的次数
def count_word(self, word_list):
word_dict = {}
for word in word_list:
if word in word_dict:
word_dict[word] += 1
else:
word_dict[word] = 1
return word_dict
# 训练模型
def train(self, train_data, train_label):
n = len(train_data)
for i in range(n):
# 获取当前文档数据和标签
data = train_data[i]
label = train_label[i]
# 更新每个分类中的文档数
if label not in self.class_count:
self.class_count[label] = 1
else:
self.class_count[label] += 1
# 更新该分类中的单词数
if label not in self.word_count:
self.word_count[label] = {}
word_dict = self.count_word(self.text_parse(data))
for word, count in word_dict.items():
if word not in self.vocab:
self.vocab.add(word)
if word not in self.word_count[label]:
self.word_count[label][word] = count
else:
self.word_count[label][word] += count
# 预测
def predict(self, data):
# 计算每个分类的概率
class_prob = {}
for label in self.class_count.keys():
class_prob[label] = math.log(self.class_count[label] / sum(self.class_count.values()))
# 计算每个单词的概率
word_prob = {}
for word in self.vocab:
word_prob[word] = {}
for label in self.class_count.keys():
if word not in self.word_count[label]:
word_prob[word][label] = 0
else:
word_prob[word][label] = math.log((self.word_count[label][word] + 1) / (sum(self.word_count[label].values()) + len(self.vocab)))
# 计算该文档属于每个分类的概率
prob = {}
word_list = self.text_parse(data)
for label in self.class_count.keys():
prob[label] = class_prob[label]
for word in word_list:
if word in self.vocab:
prob[label] += word_prob[word][label]
# 返回概率最大的分类
return max(prob, key=prob.get)
# 加载训练数据
train_data = []
train_label = []
for file_name in os.listdir('train_data'):
with open(os.path.join('train_data', file_name), 'r', encoding='utf-8') as f:
train_data.append(f.read())
train_label.append(file_name.split('.')[0])
# 训练模型
nb = NaiveBayes()
nb.train(train_data, train_label)
# 加载测试数据
test_data = []
test_label = []
for file_name in os.listdir('test_data'):
with open(os.path.join('test_data', file_name), 'r', encoding='utf-8') as f:
test_data.append(f.read())
test_label.append(file_name.split('.')[0])
# 测试模型
correct = 0
for i in range(len(test_data)):
result = nb.predict(test_data[i])
if result == test_label[i]:
correct += 1
accuracy = correct / len(test_data)
print('Accuracy:', accuracy)
```
上述代码中,我们首先定义了一个`NaiveBayes`类,实现了训练和预测方法。在训练过程中,我们先将每个文档拆分为单词列表,然后统计每个分类中的单词数和文档数。在预测过程中,我们计算每个分类的概率和每个单词的概率,然后根据朴素贝叶斯公式计算该文档属于每个分类的概率,最终返回概率最大的分类。
在示例代码中,我们将训练数据和测试数据分别存储在`train_data`和`test_data`文件夹中,每个文档的文件名为其所属分类的名称。我们首先加载训练数据,训练模型,然后加载测试数据,测试模型的准确率。
需要注意的是,该代码仅供参考,实际情况中可能需要对其进行修改和优化,以适应不同的数据集和需求。
阅读全文
相关推荐
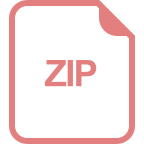
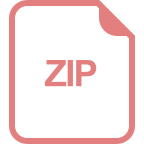
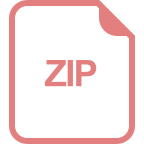
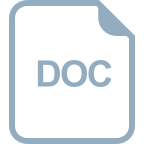
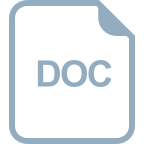
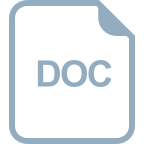
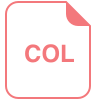
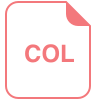
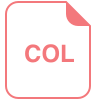
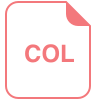
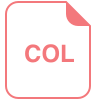
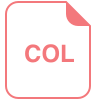
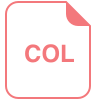
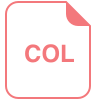
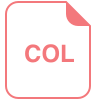
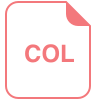
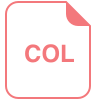