rrt matlab算法
时间: 2023-08-25 13:07:08 浏览: 94
RRT (Rapidly-exploring Random Tree) 是一种基于树结构的路径规划算法,它的目的是在高维空间中搜索可行路径。RRT 算法的基本思想是,从起点开始,以一定的概率随机选择新的节点,然后将该节点连接到已有的树中最近的节点,最终形成一颗树状结构,从而找到一条从起点到目标点的路径。
在 MATLAB 中实现 RRT 算法可以参考以下步骤:
1. 定义起点和目标点,并将起点添加到树中。
2. 设置随机点生成的次数,根据设定的概率随机生成新的节点。
3. 针对每个新节点,找到树中距离最近的节点,并计算两点之间的距离。
4. 判断新节点与距离最近的节点之间是否存在障碍物,如果存在,则舍弃该节点。
5. 如果不存在障碍物,则将新节点添加到树中,并将新节点与距离最近的节点之间的连线加入到树中。
6. 如果新节点距离目标点足够近,则停止搜索,否则继续生成新节点。
7. 最终得到的树形结构就是搜索到的路径。
需要注意的是,在实现 RRT 算法时,需要根据具体场景进行参数的调整,以保证算法的效率和精度。
相关问题
rrt matlab算法,rrt算法matlab代码
以下是基于 RRT(Rapidly-exploring Random Tree)算法的 Matlab 代码,用于构建机器人路径规划:
```matlab
clear all; close all; clc
% Environment and robot parameters
xMin = 0;
xMax = 10;
yMin = 0;
yMax = 10;
obs = [4 4 1; 6 6 1];
robotLength = 0.5;
robotWidth = 0.5;
% Start and goal positions
startPos = [1,1];
goalPos = [9,9];
% RRT parameters
maxNodes = 5000;
stepSize = 0.5;
nodes(1).x = startPos(1);
nodes(1).y = startPos(2);
nodes(1).parent = 0;
for i = 2:maxNodes
x = rand*(xMax-xMin) + xMin;
y = rand*(yMax-yMin) + yMin;
node = [x,y];
% Check if node is inside an obstacle
insideObs = 0;
for j = 1:size(obs,1)
if sqrt((node(1)-obs(j,1))^2 + (node(2)-obs(j,2))^2) < obs(j,3)
insideObs = 1;
break;
end
end
if insideObs == 1
continue;
end
% Find the nearest node
dist = inf;
nearestNode = 0;
for j = 1:length(nodes)
d = sqrt((node(1)-nodes(j).x)^2 + (node(2)-nodes(j).y)^2);
if d < dist
dist = d;
nearestNode = j;
end
end
% Check if there is a direct path between the nearest node and the new node
theta = atan2(node(2)-nodes(nearestNode).y, node(1)-nodes(nearestNode).x);
xTest = nodes(nearestNode).x + stepSize*cos(theta);
yTest = nodes(nearestNode).y + stepSize*sin(theta);
if sqrt((xTest-node(1))^2 + (yTest-node(2))^2) > stepSize
continue;
end
% Check if the direct path is inside an obstacle
insideObs = 0;
for j = 1:size(obs,1)
if sqrt((xTest-obs(j,1))^2 + (yTest-obs(j,2))^2) < obs(j,3)
insideObs = 1;
break;
end
end
if insideObs == 1
continue;
end
% Add the new node to the tree
nodes(end+1).x = xTest;
nodes(end).y = yTest;
nodes(end).parent = nearestNode;
% Check if the goal has been reached
if sqrt((nodes(end).x-goalPos(1))^2 + (nodes(end).y-goalPos(2))^2) < stepSize
break;
end
end
% Plot the obstacles
figure(1)
hold on
axis([xMin xMax yMin yMax])
for i = 1:size(obs,1)
rectangle('Position',[obs(i,1)-obs(i,3), obs(i,2)-obs(i,3), 2*obs(i,3), 2*obs(i,3)], 'Curvature', [1,1], 'FaceColor', 'r')
end
% Plot the tree
for i = 2:length(nodes)
x = [nodes(i).x, nodes(nodes(i).parent).x];
y = [nodes(i).y, nodes(nodes(i).parent).y];
plot(x,y,'k')
end
% Find the path from start to goal
path = [nodes(end).x, nodes(end).y];
while nodes(end).parent ~= 0
endNode = nodes(end);
path = [endNode.x, endNode.y; path];
endNode = nodes(endNode.parent);
end
path = [startPos; path];
% Plot the path
plot(path(:,1), path(:,2), 'b', 'LineWidth', 2)
```
这段代码会生成一个基于 RRT 算法的路径规划过程,其中包括环境参数、机器人参数、起始位置、目标位置和 RRT 参数。代码将生成一个随机树,以搜索从起始位置到目标位置的路径。最终,该代码会绘制出路径和环境。
rrt*算法matlab
RRT*算法是一种用于路径规划的算法,它在RRT算法的基础上进行了改进。RRT*算法的MATLAB实现中包含了几个函数。其中,collision_check函数用于检测新节点pnew与最近节点p_nearest之间是否存在碰撞。该函数通过遍历障碍物列表obstacle_list,计算新节点与每个障碍物的距离,并判断是否小于障碍物的半径,如果小于则返回碰撞标志collisionflag为1,表示存在碰撞。[1]
另外,FindNearest函数用于找到节点树中距离随机采样点p_rand最近的节点。该函数通过计算p_rand与每个节点的距离,找到距离最小的节点的索引minID,并返回该索引。如果存在多个距离最小的节点,只取第一个返回。[2]
而Sample函数用于生成随机采样点X_rand。该函数通过判断一个随机数是否小于0.5来确定是进行均匀采样还是使用目标点作为采样点。如果小于0.5,则在地图的范围内随机生成一个点作为采样点;否则,采样点为目标点。[3]
综上所述,RRT*算法的MATLAB实现中包含了collision_check、FindNearest和Sample等函数,它们分别用于检测碰撞、寻找最近节点和生成随机采样点。
阅读全文
相关推荐
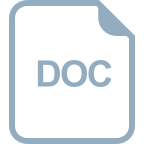
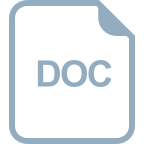
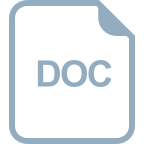
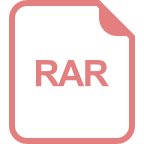
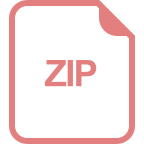
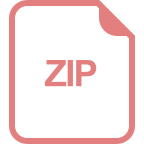









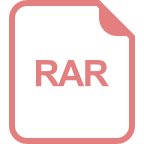