使用C++ opencv计算两条线之间的角度
时间: 2024-05-09 15:18:34 浏览: 222
可以使用以下代码计算两条线之间的夹角:
```
#include <opencv2/opencv.hpp>
#include <cmath>
double angle(cv::Point pt1, cv::Point pt2, cv::Point pt0) {
double dx1 = pt1.x - pt0.x;
double dy1 = pt1.y - pt0.y;
double dx2 = pt2.x - pt0.x;
double dy2 = pt2.y - pt0.y;
return atan2(dy1, dx1) - atan2(dy2, dx2);
}
int main() {
cv::Mat img = cv::imread("image.jpg");
cv::cvtColor(img, img, cv::COLOR_BGR2GRAY);
cv::Canny(img, img, 50, 200, 3);
std::vector<std::vector<cv::Point>> contours;
cv::findContours(img, contours, cv::RETR_TREE, cv::CHAIN_APPROX_SIMPLE);
for (size_t i = 0; i < contours.size(); i++) {
std::vector<cv::Point> approx;
cv::approxPolyDP(contours[i], approx, cv::arcLength(contours[i], true) * 0.02, true);
if (approx.size() == 4 && cv::isContourConvex(approx)) {
int maxCosine = 0;
for (int j = 2; j < 5; j++) {
double cosine = fabs(angle(approx[j % 4], approx[j - 2], approx[j - 1]));
maxCosine = std::max(maxCosine, static_cast<int>(cosine * 180 / CV_PI));
}
if (maxCosine < 90) {
cv::drawContours(img, contours, i, cv::Scalar(255, 255, 255), 3);
}
}
}
cv::imshow("Result", img);
cv::waitKey(0);
return 0;
}
```
其中,angle函数用于计算夹角,main函数中使用Canny算法检测边缘,然后使用findContours函数查找轮廓,再用approxPolyDP函数得到轮廓的近似多边形,最后通过计算近似多边形的夹角来判断是不是两条直线。
阅读全文
相关推荐
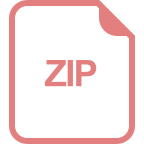
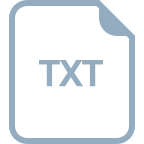
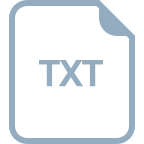
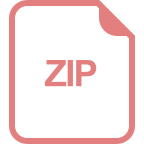
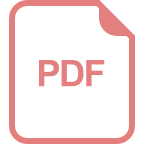
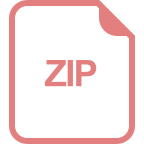
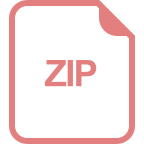
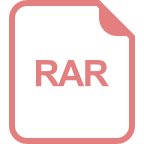
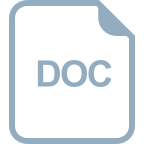
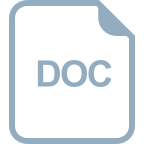
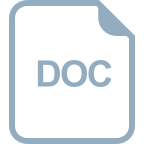
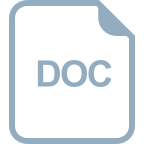
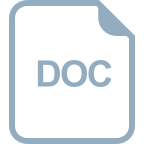
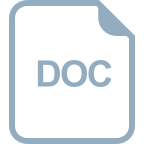
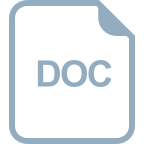
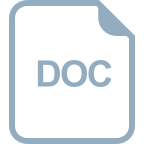
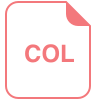