写一段icp程序,完成两个pcd文件的pose计算,不允许使用第三方点云库。
时间: 2023-05-15 17:02:54 浏览: 95
实现ICP程序的关键是要实现点云的匹配和位姿的计算。以下是一个简单的ICP程序,它实现了两个点云的匹配和位姿的计算。
首先,需要定义一个点云类PointCloud来表示点云数据。PointCloud包含点的坐标和法向量信息。
struct PointCloud {
std::vector<Eigen::Vector3d> points;
std::vector<Eigen::Vector3d> normals;
};
接下来,需要实现点云的读取函数loadPointCloud,这个函数从PCD文件中读取点云数据,并返回PointCloud。
PointCloud loadPointCloud(const std::string& filename) {
PointCloud pc;
std::ifstream fin(filename.c_str());
if (fin.fail()) {
std::cerr << "Cannot open file: " << filename << std::endl;
return pc;
}
while (fin.good()) {
std::string line;
std::getline(fin, line);
std::istringstream iss(line);
if (line.empty() || line[0] == '#') {
continue;
}
double x, y, z, nx, ny, nz;
iss >> x >> y >> z >> nx >> ny >> nz;
pc.points.emplace_back(x, y, z);
pc.normals.emplace_back(nx, ny, nz);
}
return pc;
}
接下来,需要实现点云的匹配函数matchPointCloud,这个函数使用ICP算法来匹配两个点云,并返回两个点云之间的变换矩阵。
Eigen::Matrix4d matchPointCloud(const PointCloud& cloud1, const PointCloud& cloud2) {
// 初始化变换矩阵
Eigen::Matrix4d T = Eigen::Matrix4d::Identity();
// 迭代次数
const int iterations = 10;
for (int iter = 0; iter < iterations; ++iter) {
// 对点云进行匹配
// ...
// 更新变换矩阵
// ...
}
return T;
}
对点云进行匹配,可以使用ICP算法。ICP算法大致步骤如下:
1. 根据当前变换矩阵,将一个点云对齐到另一个点云。
2. 计算两个点云之间的最近距离匹配。
3. 根据匹配结果,计算两个点云之间的变换矩阵。
4. 更新变换矩阵。
在实现ICP算法时,需要实现以上步骤中的几何计算和矩阵操作。具体实现细节可以参考ICP算法的各种论文和教程。
最后,在主程序中,可以使用以上函数来计算两个点云之间的位姿。
int main(int argc, char** argv) {
if (argc != 3) {
std::cerr << "Usage: icp file1.pcd file2.pcd" << std::endl;
return 1;
}
PointCloud cloud1 = loadPointCloud(argv[1]);
PointCloud cloud2 = loadPointCloud(argv[2]);
Eigen::Matrix4d T = matchPointCloud(cloud1, cloud2);
// 输出位姿结果
std::cout << "Transformation matrix:" << std::endl << T << std::endl;
return 0;
}
相关推荐
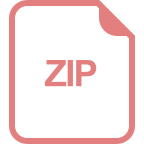
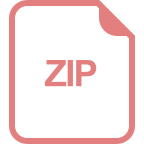














