#include<iostream> #include<fstream> using namespace std; int main() { fstream iofile; iofile.open("test.txt", ios::out); iofile << "12345#1#2" << "#3\n"; iofile.close(); iofile.open("test.txt", ios::in); int i; while (iofile >> i) { if (i >= 1 && i <= 3) cout << '*'; else cout << i; } cout << endl; iofile.close(); return 0; }
时间: 2024-01-14 20:02:31 浏览: 19
以上代码的执行结果为:
```
12345*1*2*3
```
代码的作用是向文件"test.txt"中写入一行字符串"12345#1#2#3\n",然后再从文件中读取整数并将它们输出到屏幕上,如果读取到的整数在1~3之间,则输出"*",否则直接输出该整数。具体的操作流程如下:
1. 定义一个`fstream`类型的对象`iofile`,并打开文件"test.txt",以供写入数据。
2. 向文件中写入一行字符串"12345#1#2#3\n",其中"#"和"\n"分别表示一个分隔符和一个换行符。由于文件以输出模式打开,因此可以使用`<<`运算符来向文件中写入数据。
3. 关闭文件。
4. 再次打开文件"test.txt",以供读取数据。
5. 使用`while`循环从文件中读取整数,并将它们输出到屏幕上。由于文件以输入模式打开,因此可以使用`>>`运算符来从文件中读取数据。由于文件中的数字之间用分隔符"#"分隔,因此可以使用`>>`运算符来忽略分隔符,只读取数字。如果读取到的数字在1~3之间,则输出"*",否则直接输出该数字。
6. 关闭文件。
7. 返回0,表示程序正常结束。
因此,最终输出的结果是:
```
12345*1*2*3
```
其中,数字1、2、3被替换成了"*"。
相关问题
以下代码的执行结果为 (1) 。 #include<iostream> #include<fstream> using namespace std; int main() { fstream iofile; iofile.open("test.txt", ios::out); iofile << "12345#1#2" << "#3\n"; iofile.close(); iofile.open("test.txt", ios::in); int i; while (iofile >> i) { if (i >= 1 && i <= 3) cout << '*'; else cout << i; } cout << endl; iofile.close(); return 0; }
以下代码的执行结果为:
```
12345*1*2*3
```
代码的作用是先将字符串"12345#1#2"写入到文件"test.txt"中,然后再从文件中读取数据,并将读取到的数据输出到屏幕上。在读取数据时,如果读取到的数据在1到3之间,则输出"*",否则直接输出读取到的数字。
具体的操作流程如下:
1. 定义一个`fstream`类型的对象`iofile`。
2. 打开文件"test.txt",并设置文件的打开模式为输出模式(`ios::out`)。
3. 使用`<<`运算符将字符串"12345#1#2"写入到文件中。
4. 关闭文件。
5. 再次打开文件"test.txt",并设置文件的打开模式为输入模式(`ios::in`)。
6. 定义一个整型变量`i`。
7. 使用`while`循环从文件中逐个读取数据,如果读取到的数据在1到3之间,则输出"*",否则直接输出读取到的数字。
8. 关闭文件。
因此,最终输出的结果是"12345*1*2*3"。
#include<iostream> #include<fstream> using namespace std; class Student { public: int num; char name[20]; }; int main() { fstream iofile("File.txt", ios::in | ios::out | ios::binary); if (!iofile) { cout << "打开失败" << endl; exit(1); } Student st[2] = { {101 ,"Tom"},{102,"Bob"} }, stunew[2]; for (int i = 0; i < 2; i++) { iofile.write((char*)&st[i], sizeof(Student)); } for (int i = 0; i < 2; i++) { iofile.seekg(i * sizeof(st[i]), ios::beg); iofile.read((char*)&stunew[i], sizeof(Student)); } strcpy(stunew[1].name, "Lux"); stunew[1].num = 103; iofile.seekp(sizeof(st[0]), ios::beg); iofile.write((char*)&stunew[1], sizeof(Student)); iofile.seekg(0, ios::beg); for (int i = 0; i < 2; i++) { iofile.read((char*)&stunew[i], sizeof(Student)); cout << "第" << i + 1 << "个同学的信息:" << stunew[i].name << ":" << stunew[i].num << endl; } iofile.close(); }代码为什么运行错误
你的代码运行错误的原因是,你在写入文件时使用了 `ios::binary` 标志,但在读取文件时没有使用该标志。这会导致在 Windows 系统上读取二进制文件时出现问题。
为了解决这个问题,你需要在读取文件时也使用 `ios::binary` 标志,像这样:
```
iofile.seekg(i * sizeof(st[i]), ios::beg);
iofile.read((char*)&stunew[i], sizeof(Student));
```
修改后的完整代码如下:
```
#include<iostream>
#include<fstream>
using namespace std;
class Student {
public:
int num;
char name[20];
};
int main() {
fstream iofile("File.txt", ios::in | ios::out | ios::binary);
if (!iofile)
{
cout << "打开失败" << endl;
exit(1);
}
Student st[2] = { {101 ,"Tom"},{102,"Bob"} }, stunew[2];
for (int i = 0; i < 2; i++)
{
iofile.write((char*)&st[i], sizeof(Student));
}
for (int i = 0; i < 2; i++)
{
iofile.seekg(i * sizeof(st[i]), ios::beg);
iofile.read((char*)&stunew[i], sizeof(Student));
}
strcpy(stunew[1].name, "Lux");
stunew[1].num = 103;
iofile.seekp(sizeof(st[0]), ios::beg);
iofile.write((char*)&stunew[1], sizeof(Student));
iofile.seekg(0, ios::beg);
for (int i = 0; i < 2; i++)
{
iofile.read((char*)&stunew[i], sizeof(Student));
cout << "第" << i + 1 << "个同学的信息:" << stunew[i].name << ":" << stunew[i].num << endl;
}
iofile.close();
return 0;
}
```
相关推荐
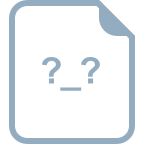












