cv2.VideoCapture(3)
时间: 2024-03-10 10:10:02 浏览: 21
cv2.VideoCapture() 是 OpenCV 中的一个函数,用于打开视频文件或摄像头,并创建一个 VideoCapture 对象。可以使用该对象来读取视频帧并进行处理。该函数的第一个参数可以是视频文件的路径或者摄像头的编号(0表示第一个摄像头,1表示第二个摄像头等等)。例如:
```
import cv2
# 打开摄像头
cap = cv2.VideoCapture(0)
while True:
# 读取帧
ret, frame = cap.read()
# 将帧显示在窗口上
cv2.imshow("Webcam", frame)
# 按下 'q' 键退出
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放摄像头并关闭窗口
cap.release()
cv2.destroyAllWindows()
```
这将打开第一个摄像头并在窗口中显示实时视频。按下“q”键退出程序。
相关问题
cv2.VideoCapture
cv2.VideoCapture是OpenCV中用于捕获视频的函数。它可以从摄像头、视频文件或网络视频流中获取帧。可以使用该函数来读取视频文件中的帧并进行处理,例如分析视频中的运动、检测和跟踪对象等。
使用cv2.VideoCapture函数时,需要传入一个参数,该参数可以是摄像头设备的编号,也可以是视频文件的路径。例如,如果要从电脑上连接的摄像头获取视频,则可以使用以下代码:
```
import cv2
cap = cv2.VideoCapture(0)
while(True):
# Capture frame-by-frame
ret, frame = cap.read()
# Display the resulting frame
cv2.imshow('frame',frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# When everything done, release the capture
cap.release()
cv2.destroyAllWindows()
```
在上面的代码中,我们使用了cv2.VideoCapture(0)来获取摄像头设备的编号为0的摄像头。然后在while循环中,使用cap.read()函数获取每一帧视频,并在窗口中显示出来。在按下“q”键后,释放摄像头并关闭所有窗口。
cv2.videocapture
cv2.VideoCapture() 是 OpenCV 中用于读取视频文件或者从摄像头采集实时视频的函数。它可以返回一个 VideoCapture 对象,该对象包含了视频文件或者摄像头的相关信息,并提供了一些方法来操作这些视频信息,例如读取每一帧、设置视频的尺寸和帧率等。
使用 cv2.VideoCapture() 读取视频文件或者摄像头时,需要传入一个参数,用于指定要读取的视频来源。参数可以是视频文件的路径、摄像头的编号(0 表示默认的摄像头,1 表示第二个摄像头,以此类推)或者 IP 地址(用于读取网络摄像头的视频流)。
例子:
```
import cv2
cap = cv2.VideoCapture('video.mp4')
while cap.isOpened():
ret, frame = cap.read()
if ret:
cv2.imshow('frame', frame)
else:
break
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
```
这段代码会打开名为 "video.mp4" 的视频文件,读取每一帧并展示在窗口中,直到按下 'q' 键退出。
相关推荐
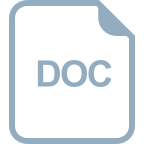
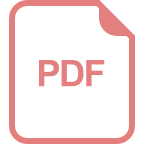
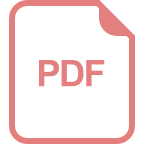










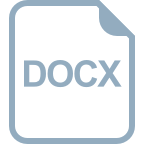