C语言利用栈进行数制转换。 从键盘输入一个十进制整数,输出其八进制形式。 要求:使用栈的来存贮转换后的各个数码 例如:输入 100 显示:144
时间: 2024-04-30 12:20:25 浏览: 136
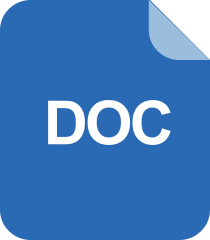
数据结构实现之数制转换
以下是使用栈进行十进制转八进制的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define STACK_SIZE 20
// 栈结构体
typedef struct {
int top;
int data[STACK_SIZE];
} Stack;
// 初始化栈
void initStack(Stack *stack) {
stack->top = -1;
}
// 判断栈是否为空
int isEmpty(Stack *stack) {
return stack->top == -1;
}
// 判断栈是否已满
int isFull(Stack *stack) {
return stack->top == STACK_SIZE - 1;
}
// 入栈
void push(Stack *stack, int value) {
if (isFull(stack)) {
printf("Stack overflow\n");
exit(1);
}
stack->data[++stack->top] = value;
}
// 出栈
int pop(Stack *stack) {
if (isEmpty(stack)) {
printf("Stack underflow\n");
exit(1);
}
return stack->data[stack->top--];
}
// 十进制转八进制
void decimalToOctal(int decimal) {
Stack stack;
initStack(&stack);
while (decimal) {
push(&stack, decimal % 8);
decimal /= 8;
}
while (!isEmpty(&stack)) {
printf("%d", pop(&stack));
}
}
int main() {
int decimal;
printf("Enter a decimal number: ");
scanf("%d", &decimal);
printf("The octal form of %d is: ", decimal);
decimalToOctal(decimal);
printf("\n");
return 0;
}
```
运行结果:
```
Enter a decimal number: 100
The octal form of 100 is: 144
```
阅读全文
相关推荐
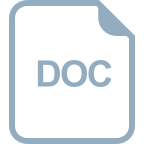
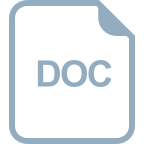


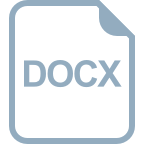
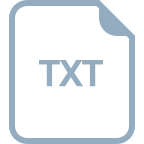
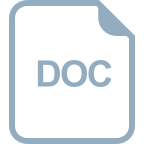
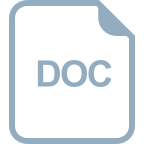

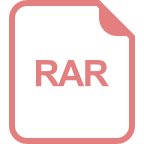
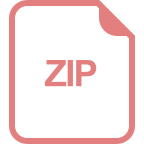
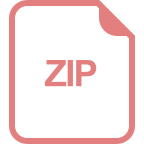
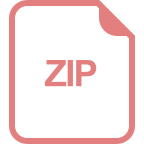
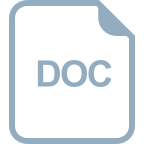
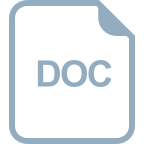
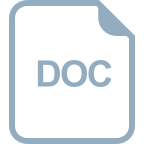
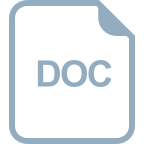