在linux中使用C语言编程贪吃蛇代码
时间: 2024-05-12 12:19:21 浏览: 94
以下是一个简单的贪吃蛇游戏代码,使用C语言编写,可以在Linux系统中运行。
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <termios.h>
#include <fcntl.h>
#define ROW 20
#define COL 40
int x, y; // 蛇头坐标
int fx, fy; // 食物坐标
int score; // 得分
int len; // 蛇长
int dir; // 方向
int map[ROW][COL]; // 地图
int getch(void)
{
struct termios oldt, newt;
int ch;
tcgetattr(STDIN_FILENO, &oldt);
newt = oldt;
newt.c_lflag &= ~(ICANON | ECHO);
tcsetattr(STDIN_FILENO, TCSANOW, &newt);
ch = getchar();
tcsetattr(STDIN_FILENO, TCSANOW, &oldt);
return ch;
}
void init() // 初始化
{
int i, j;
x = ROW / 2;
y = COL / 2;
score = 0;
len = 1;
dir = 1;
map[x][y] = len;
fx = rand() % ROW;
fy = rand() % COL;
for (i = 0; i < ROW; i++) {
for (j = 0; j < COL; j++) {
if (i == 0 || i == ROW - 1 || j == 0 || j == COL - 1) {
map[i][j] = -1;
}
else {
map[i][j] = 0;
}
}
}
map[fx][fy] = -2;
}
void draw() // 绘制
{
system("clear");
int i, j;
for (i = 0; i < ROW; i++) {
for (j = 0; j < COL; j++) {
if (map[i][j] == -1) {
printf("#");
}
else if (map[i][j] == 0) {
printf(" ");
}
else if (map[i][j] == -2) {
printf("$");
}
else {
printf("*");
}
}
printf("\n");
}
printf("Score: %d\n", score);
}
void update() // 更新
{
int i, j;
int nx = x, ny = y;
switch (dir) {
case 1: // 上
nx--;
break;
case 2: // 下
nx++;
break;
case 3: // 左
ny--;
break;
case 4: // 右
ny++;
break;
}
if (nx == fx && ny == fy) { // 吃到食物
len++;
score += 10;
fx = rand() % ROW;
fy = rand() % COL;
}
else if (map[nx][ny] != 0) { // 撞到自己
printf("Game Over!\n");
exit(0);
}
else { // 移动
for (i = 0; i < ROW; i++) {
for (j = 0; j < COL; j++) {
if (map[i][j] > 0) {
map[i][j]--;
}
}
}
}
map[x][y] = len;
x = nx;
y = ny;
map[x][y] = len + 1;
}
int main()
{
init();
while (1) {
draw();
usleep(100000);
if (kbhit()) { // 按键
int ch = getch();
if (ch == 27) { // 方向键
ch = getch();
if (ch == 65 && dir != 2) {
dir = 1;
}
else if (ch == 66 && dir != 1) {
dir = 2;
}
else if (ch == 68 && dir != 4) {
dir = 3;
}
else if (ch == 67 && dir != 3) {
dir = 4;
}
}
else if (ch == 'q') { // 退出
exit(0);
}
}
update();
}
return 0;
}
```
注意:该代码使用了Linux特有的头文件和函数,无法在Windows等其他操作系统中编译运行。
阅读全文
相关推荐
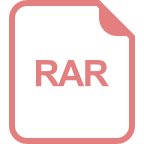
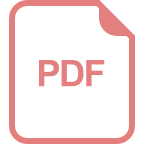
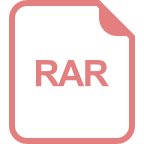
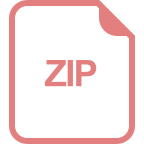
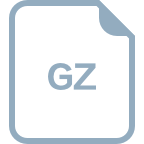
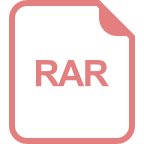
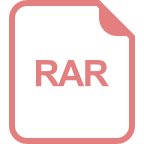
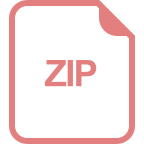
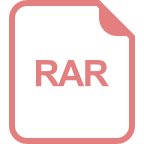
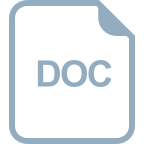
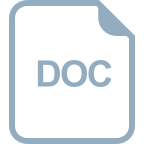
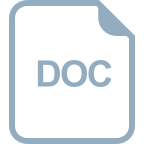

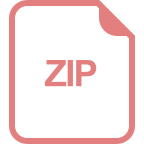
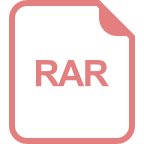
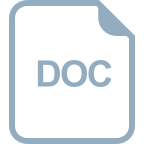
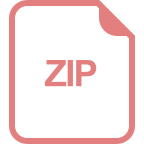
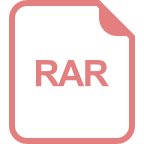