使用随机游走算法分割图像(python语言)
时间: 2023-10-12 19:14:38 浏览: 47
随机游走算法可以用来进行图像分割,其基本思想是通过在图像中随机游走来确定像素点所属的区域。具体流程如下:
1. 定义随机游走的步长和游走的次数。
2. 随机选择一个像素点作为起点。
3. 从起点开始进行随机游走,每次按照随机方向前进一个步长,并将当前像素点标记为该区域的一部分。
4. 重复进行步骤3指定次数,得到一个区域。
5. 选择另一个未标记的像素点作为起点,重复步骤3-4直到所有像素点都被标记为一个区域。
以下是一个使用随机游走算法进行图像分割的Python示例代码:
```python
import numpy as np
from PIL import Image
def random_walk(image, step_size, num_iterations):
"""
随机游走算法
:param image: 待分割的图像,PIL Image对象
:param step_size: 游走的步长
:param num_iterations: 游走的次数
:return: 分割结果,Numpy数组
"""
# 将图像转换为灰度图像
gray = image.convert('L')
# 获取图像的宽度和高度
width, height = gray.size
# 初始化标记矩阵,所有像素点都标记为未访问
visited = np.zeros((height, width), dtype=np.bool)
# 初始化分割结果矩阵,所有像素点都标记为0
result = np.zeros((height, width), dtype=np.int)
# 定义随机方向数组
directions = [(-1, 0), (0, -1), (1, 0), (0, 1)]
# 随机选择一个起点
start_x, start_y = np.random.randint(width), np.random.randint(height)
# 重复进行游走,直到所有像素点都被标记为一个区域
while not visited.all():
# 如果当前起点已被访问,则选择另一个起点
if visited[start_y, start_x]:
start_x, start_y = np.random.randint(width), np.random.randint(height)
continue
# 标记当前像素点已被访问
visited[start_y, start_x] = True
# 进行随机游走
for i in range(num_iterations):
# 随机选择一个方向
dx, dy = directions[np.random.randint(4)]
# 计算新的像素点坐标
new_x, new_y = start_x + dx * step_size, start_y + dy * step_size
# 如果新的像素点超出图像边界,则选择另一个方向
if new_x < 0 or new_x >= width or new_y < 0 or new_y >= height:
continue
# 如果新的像素点已被访问,则选择另一个方向
if visited[new_y, new_x]:
continue
# 标记新的像素点已被访问
visited[new_y, new_x] = True
# 将新的像素点标记为当前区域的一部分
result[new_y, new_x] = result[start_y, start_x]
# 选择一个未被标记的像素点作为下一个起点
unvisited_indices = np.transpose(np.nonzero(~visited))
if len(unvisited_indices) > 0:
start_y, start_x = unvisited_indices[np.random.randint(len(unvisited_indices))]
# 选择一个未被标记的区域作为下一个区域的标记
if len(np.unique(result)) == 1:
result[start_y, start_x] = 1
else:
unvisited_region_indices = np.transpose(np.nonzero(result == 0))
if len(unvisited_region_indices) > 0:
result[start_y, start_x] = np.unique(result[unvisited_region_indices])[0]
return result
# 测试代码
if __name__ == '__main__':
# 读取图像文件
image = Image.open('test.jpg')
# 进行图像分割
result = random_walk(image, 10, 100)
# 将分割结果保存为图像文件
result_image = Image.fromarray((result * 255 / np.max(result)).astype(np.uint8))
result_image.save('result.jpg')
```
在上述代码中,我们首先将图像转换为灰度图像,并初始化标记矩阵和分割结果矩阵。然后随机选择一个起点,并进行随机游走,将经过的像素点标记为当前区域的一部分,重复进行直到所有像素点都被标记为一个区域。选择下一个起点和下一个区域的标记的方法在代码中有详细的注释。最后将分割结果保存为图像文件。
相关推荐
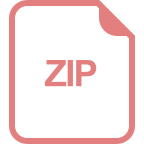
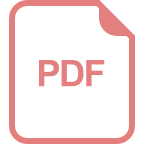
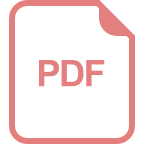














