定义日期类,重载 “++”和“--”运算符,使之能处理两个日期类对象自增和自减运算。(使用c++)
时间: 2024-02-23 14:59:57 浏览: 80
下面是一个简单的日期类实现,包括两个日期对象的自增和自减运算符重载:
```c++
#include <iostream>
using namespace std;
class Date {
private:
int year, month, day;
public:
Date(int y, int m, int d) {
year = y;
month = m;
day = d;
}
int getYear() const {
return year;
}
int getMonth() const {
return month;
}
int getDay() const {
return day;
}
Date& operator++() {
day++;
if (day > daysInMonth()) {
day = 1;
month++;
if (month > 12) {
month = 1;
year++;
}
}
return *this;
}
Date& operator--() {
day--;
if (day < 1) {
month--;
if (month < 1) {
month = 12;
year--;
}
day = daysInMonth();
}
return *this;
}
private:
int daysInMonth() const {
switch (month) {
case 2:
if ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)) {
return 29;
} else {
return 28;
}
case 4:
case 6:
case 9:
case 11:
return 30;
default:
return 31;
}
}
};
ostream& operator<<(ostream& os, const Date& date) {
os << date.getYear() << "-" << date.getMonth() << "-" << date.getDay();
return os;
}
int main() {
Date d1(2021, 10, 1);
Date d2(2021, 10, 31);
cout << "d1: " << d1 << endl;
cout << "d2: " << d2 << endl;
++d1;
--d2;
cout << "d1++: " << d1 << endl;
cout << "d2--: " << d2 << endl;
return 0;
}
```
在上面的代码中,我们定义了一个 `Date` 类,它具有年、月、日的属性,并实现了自增和自减运算符,这些运算符可以自动处理日期的溢出和借位。我们还重载了输出运算符 `<<`,以便更方便地打印日期对象。在 `main` 函数中,我们创建了两个日期对象 `d1` 和 `d2`,然后对它们进行了自增和自减操作,并打印了结果。
阅读全文
相关推荐
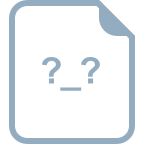
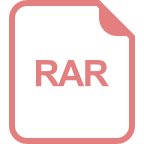
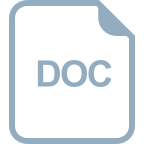















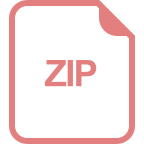