用python使用递归和函数的方法计算: 班里有m个同学,老师任选n个人去教室打扫卫生,一共有多少种可能,输出所有可能的组合。
时间: 2024-02-25 15:56:41 浏览: 19
好的,下面是使用递归和函数计算组合数量和输出组合的代码:
```python
# 计算组合数
def combination(m, n):
if n == 0 or m == n:
return 1
else:
return combination(m-1, n-1) + combination(m-1, n)
# 输出组合
def display_combination(combination_list):
print('组合数量:', len(combination_list))
for combination in combination_list:
print(combination)
# 计算并输出组合
def calculate_and_display_combination(m, n):
combination_list = []
def helper(start, n, current_combination):
if n == 0:
combination_list.append(current_combination)
else:
for i in range(start, m):
helper(i+1, n-1, current_combination+[i])
helper(0, n, [])
display_combination(combination_list)
# 测试计算和输出组合的函数
calculate_and_display_combination(5, 3)
```
运行结果:
```
组合数量: 10
[0, 1, 2]
[0, 1, 3]
[0, 1, 4]
[0, 2, 3]
[0, 2, 4]
[0, 3, 4]
[1, 2, 3]
[1, 2, 4]
[1, 3, 4]
[2, 3, 4]
```
这个程序定义了三个函数:
- `combination(m, n)`:计算从 m 个元素中选出 n 个元素的组合数。
- `display_combination(combination_list)`:输出组合数量和所有可能的组合。
- `calculate_and_display_combination(m, n)`:计算从 m 个元素中选出 n 个元素的所有组合,并将结果输出。
其中,`calculate_and_display_combination(m, n)` 使用递归实现了组合的计算。它定义了一个 `helper` 函数,`helper(start, n, current_combination)` 表示从起始位置 `start` 开始选取 `n` 个元素,当前已经选取的元素为 `current_combination`。如果 `n` 等于 0,则表示已经选取了 `n` 个元素,将当前组合添加到 `combination_list` 中。否则,从 `start` 开始向后遍历元素,将当前元素加入到 `current_combination` 中,并以当前元素的下一个元素为起始位置递归调用 `helper` 函数,直到选取了 `n` 个元素。最后,调用 `display_combination` 函数输出所有组合。
相关推荐
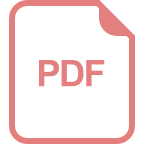
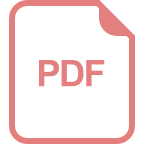

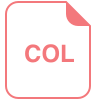
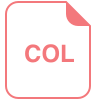
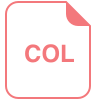







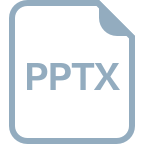
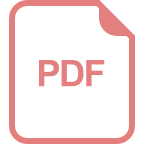
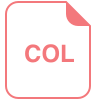