用python将pcd文件转成TXT文件的代码
时间: 2023-04-05 07:03:36 浏览: 175
可以使用以下Python代码将pcd文件转换为txt文件:
```python
import numpy as np
def pcd_to_txt(pcd_file, txt_file):
with open(pcd_file, 'r') as f:
lines = f.readlines()
data_start = False
data = []
for line in lines:
if data_start:
data.append(line.strip().split())
if line.startswith('DATA'):
data_start = True
data = np.array(data).astype(np.float32)
np.savetxt(txt_file, data, delimiter=' ', fmt='%.6f')
```
其中,`pcd_file`是输入的pcd文件路径,`txt_file`是输出的txt文件路径。使用`np.savetxt`函数将数据保存为txt文件。
相关问题
用python读取pcd文件转成TXT文件的代码
以下是用Python读取pcd文件并转换为TXT文件的代码:
```python
import numpy as np
def read_pcd_file(file_path):
with open(file_path, 'r') as f:
lines = f.readlines()
data_start = False
data = []
for line in lines:
if line.startswith('DATA'):
data_start = True
continue
if data_start:
data.append(line.strip().split())
return np.array(data, dtype=np.float32)
def write_txt_file(data, file_path):
with open(file_path, 'w') as f:
for row in data:
f.write(' '.join([str(x) for x in row]) + '\n')
if __name__ == '__main__':
pcd_file_path = 'example.pcd'
txt_file_path = 'example.txt'
data = read_pcd_file(pcd_file_path)
write_txt_file(data, txt_file_path)
```
请注意,这只是一个示例代码,实际上,读取和写入文件的方式可能因pcd文件的格式而异。
python把bin文件转换成pcd文件
你可以使用Python中的open3d库来读取bin文件并将其转换为pcd文件。以下是一个示例代码:
```python
import open3d as o3d
import numpy as np
# 读取bin文件
point_cloud = np.fromfile("input.bin", dtype=np.float32).reshape(-1, 4)
# 将numpy数组转换为Open3D点云对象
pcd = o3d.geometry.PointCloud()
pcd.points = o3d.utility.Vector3dVector(point_cloud[:, :3])
# 保存为pcd文件
o3d.io.write_point_cloud("output.pcd", pcd)
```
请注意,此代码假定bin文件中每个点由x、y、z和intensity四个浮点数组成。如果您的bin文件格式不同,您需要相应地更改代码。
相关推荐
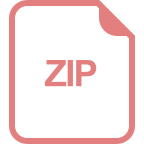
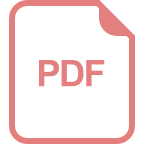












