使用CompletableFuture写一个代码案例
时间: 2023-10-18 15:15:45 浏览: 88
好的,这里给您提供一个CompletableFuture的代码案例:
```java
import java.util.concurrent.CompletableFuture;
public class CompletableFutureExample {
public static void main(String[] args) {
// 创建一个CompletableFuture对象,将结果设置为字符串"Hello"
CompletableFuture<String> future = CompletableFuture.completedFuture("Hello");
// 使用thenApply方法对结果进行转换
CompletableFuture<String> future2 = future.thenApply(s -> s + " World");
// 使用thenAccept方法对结果进行消费
future2.thenAccept(System.out::println);
// 创建一个新的CompletableFuture对象,并使用supplyAsync方法执行异步任务
CompletableFuture<String> future3 = CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
return "Async Task";
});
// 使用thenCompose方法对结果进行转换
CompletableFuture<String> future4 = future.thenCompose(s -> future3);
// 使用exceptionally方法处理异常情况
CompletableFuture<String> future5 = CompletableFuture.supplyAsync(() -> {
throw new RuntimeException("Exceptional Task");
}).exceptionally(e -> "Handled Exception: " + e.getMessage());
// 使用allOf方法等待多个CompletableFuture对象执行完毕
CompletableFuture<Void> future6 = CompletableFuture.allOf(future, future3, future4, future5);
// 使用join方法等待CompletableFuture对象执行完毕,并获取结果
try {
future6.join();
System.out.println("All tasks completed successfully!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
这个案例中,我们创建了多个CompletableFuture对象,并对它们进行了不同的操作,包括转换、消费、处理异常等。最后使用allOf方法等待多个CompletableFuture对象执行完毕,并使用join方法获取结果。
阅读全文
相关推荐
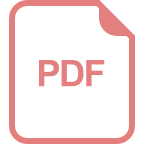
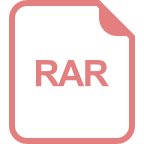
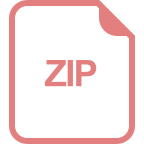










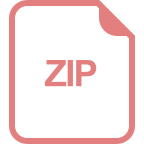
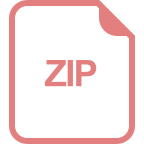
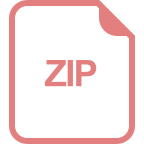
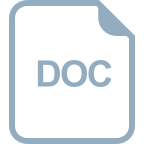
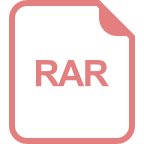
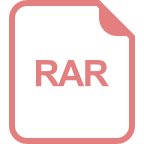