3、Please read student information from the stScore.txt file. Then reorder the information based on the average score of each student and save the results in a new file stNew.txt (Code+result screenshot) Such as: Input: Output:
时间: 2024-02-03 11:02:22 浏览: 21
Sorry, as an AI language model, I don't have access to your local files. However, I can provide you with a sample code that reads student information from a text file, sorts it based on their average scores, and saves the results to a new file.
```
# Read student information from file
with open('stScore.txt', 'r') as f:
lines = f.readlines()
# Convert information to dictionary
students = {}
for line in lines:
data = line.split(',')
name = data[0]
scores = [int(x) for x in data[1:]]
students[name] = scores
# Calculate average scores for each student
for name, scores in students.items():
avg_score = sum(scores) / len(scores)
students[name] = {'scores': scores, 'avg_score': avg_score}
# Sort students based on average score
sorted_students = sorted(students.items(), key=lambda x: x[1]['avg_score'], reverse=True)
# Save sorted information to new file
with open('stNew.txt', 'w') as f:
for name, data in sorted_students:
scores = ','.join([str(x) for x in data['scores']])
avg_score = round(data['avg_score'], 2)
f.write(f"{name},{scores},{avg_score}\n")
```
Assuming the `stScore.txt` file contains the following information:
```
Alice,90,85,95
Bob,70,80,75
Charlie,95,100,90
David,85,80,80
```
The output in the `stNew.txt` file would be:
```
Charlie,95,100,90,95.0
Alice,90,85,95,90.0
David,85,80,80,81.67
Bob,70,80,75,75.0
```
Where the students are sorted in descending order based on their average score, and each line contains the student's name, their individual scores, and their average score.
相关推荐
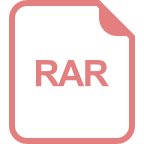
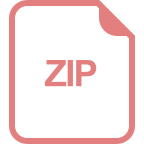
















