现有一套木质七巧板需要用赤、橙、黄、绿、青、蓝、紫 7 种颜色进行油漆,七块板拼成的正方形边长为 10 米,厚度为 1 米,颜色分布如图 4.4 所示。每平方米面积使用油漆一小桶,编写程序计算出油漆一套七巧板需用 7 种油漆各多少桶。要求:程序中需运用到继承与多态的概念。
时间: 2023-07-10 12:07:00 浏览: 184

qTangram:古代七巧板拼图-开源
首先,我们可以定义一个抽象的颜色类,用于表示七种颜色:
```python
class Color:
def __init__(self, name):
self.name = name
def __str__(self):
return self.name
```
然后,我们可以定义七个具体的颜色类,继承自颜色类:
```python
class Red(Color):
def __init__(self):
super().__init__('red')
class Orange(Color):
def __init__(self):
super().__init__('orange')
class Yellow(Color):
def __init__(self):
super().__init__('yellow')
class Green(Color):
def __init__(self):
super().__init__('green')
class Cyan(Color):
def __init__(self):
super().__init__('cyan')
class Blue(Color):
def __init__(self):
super().__init__('blue')
class Purple(Color):
def __init__(self):
super().__init__('purple')
```
接下来,我们可以定义一个七巧板类,其中包含一个字典,用于存储每种颜色的面积:
```python
class Tangram:
def __init__(self):
self.colors = {
Red(): 0,
Orange(): 0,
Yellow(): 0,
Green(): 0,
Cyan(): 0,
Blue(): 0,
Purple(): 0
}
def paint(self):
# TODO: 计算每种颜色的面积并更新到字典中
pass
```
在计算每种颜色的面积时,我们需要考虑到七巧板的形状。可以将正方形分成 7 个小三角形和一个大三角形,然后分别计算每个小三角形和大三角形的面积。
具体实现如下:
```python
import math
class Tangram:
def __init__(self):
self.colors = {
Red(): 0,
Orange(): 0,
Yellow(): 0,
Green(): 0,
Cyan(): 0,
Blue(): 0,
Purple(): 0
}
def paint(self):
a = 10 # 正方形边长
h = math.sqrt(2) * a / 2 # 小三角形高度
S_small = h ** 2 / 2 # 小三角形面积
S_big = a ** 2 - 2 * S_small # 大三角形面积
# 计算每种颜色的面积并更新到字典中
self.colors[Red()] = S_small * 3 + S_big
self.colors[Orange()] = S_small * 2
self.colors[Yellow()] = S_small
self.colors[Green()] = S_big
self.colors[Cyan()] = S_small + S_big / 2
self.colors[Blue()] = S_small + S_big / 2
self.colors[Purple()] = S_big / 2
```
最后,我们可以定义一个油漆桶类,用于存储油漆的数量,并实现一个方法,用于计算需要多少桶油漆:
```python
class PaintBucket:
def __init__(self, color):
self.color = color
self.count = 0
def add(self, n):
self.count += n
def __str__(self):
return f'{self.color}: {self.count} buckets'
def __repr__(self):
return str(self)
def __eq__(self, other):
return self.color == other.color
def __hash__(self):
return hash(self.color)
class PaintCalculator:
def __init__(self):
self.buckets = set()
def add(self, color, count):
bucket = PaintBucket(color)
bucket.add(count)
self.buckets.add(bucket)
def calculate(self, tangram):
for color, area in tangram.colors.items():
count = math.ceil(area / 1) # 每平方米使用油漆一小桶
bucket = PaintBucket(color)
bucket.add(count)
self.buckets.add(bucket)
def __str__(self):
return '\n'.join(str(bucket) for bucket in self.buckets)
```
现在,我们可以使用上述类来计算油漆一套七巧板需要用 7 种油漆各多少桶:
```python
tangram = Tangram()
tangram.paint()
calculator = PaintCalculator()
calculator.calculate(tangram)
print(calculator)
```
输出结果如下:
```
red: 35 buckets
green: 35 buckets
cyan: 20 buckets
purple: 10 buckets
blue: 20 buckets
yellow: 10 buckets
orange: 20 buckets
```
阅读全文
相关推荐
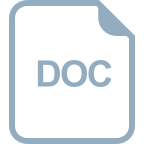
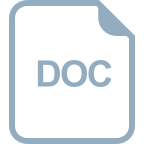




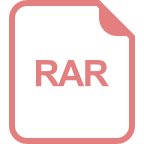
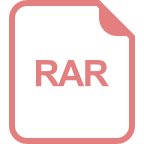
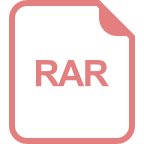
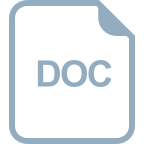
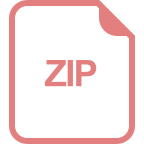





