Counter函数取出现次数最多的
时间: 2023-08-09 10:04:15 浏览: 69
可以使用Python内置的Counter函数来取出现次数最多的元素。Counter函数可以统计一个可迭代对象中每个元素出现的次数,返回一个字典,其中键为元素,值为出现次数。可以通过调用most_common()方法,返回出现次数最多的元素及其出现次数。
下面是一个例子:
```python
from collections import Counter
lst = [1, 2, 3, 1, 2, 1, 4, 5, 4, 1]
cnt = Counter(lst)
most_common = cnt.most_common(1) # 取出现次数最多的元素及其出现次数
print(most_common) # 输出:[(1, 4)]
```
上述代码中,我们首先定义了一个列表lst,然后使用Counter函数统计每个元素出现的次数,得到了一个字典cnt。然后,我们调用most_common()方法,传入参数1,即取出现次数最多的元素及其出现次数。最后,输出结果为[(1, 4)],表示元素1出现了4次,是出现次数最多的元素。
相关问题
新增函数,统计出现次数最多的字母,返回结果
好的,可以使用Python内置的collections模块中的Counter类来实现。以下是实现代码:
```python
from collections import Counter
def most_common_letter(string):
# 将字符串中的字母转换成小写,并过滤掉非字母字符
letters = [c.lower() for c in string if c.isalpha()]
# 使用Counter类统计字母出现次数
counter = Counter(letters)
# 获取出现次数最多的字母及其出现次数
most_common = counter.most_common(1)
if most_common:
return most_common[0]
else:
return None
```
在上面的代码中,我们首先通过列表推导式将字符串中的字母转换成小写,并过滤掉非字母字符。然后使用Counter类统计字母出现次数,再使用Counter类的most_common方法获取出现次数最多的字母及其出现次数。最后返回结果即可。
调用这个函数,可以得到如下结果:
```python
>>> most_common_letter("Hello, world!")
('l', 3)
>>> most_common_letter("The quick brown fox jumps over the lazy dog")
('o', 4)
>>> most_common_letter("12345")
None
```
需要注意的是,如果字符串中没有字母,函数将返回None。
求解序列中出现次数最多
可以使用 Python 中的 Counter 来统计序列中每个元素出现的次数,然后返回出现次数最多的元素。
示例代码如下:
``` python
from collections import Counter
def most_common(seq):
"""返回序列中出现次数最多的元素"""
counter = Counter(seq)
return counter.most_common(1)[0][0] # 返回出现次数最多的元素
# 示例
seq = [1, 2, 3, 2, 3, 3, 4, 5, 5]
print(most_common(seq)) # 输出 3
```
在示例中,序列中出现次数最多的是 3,因此函数返回 3。
阅读全文
相关推荐
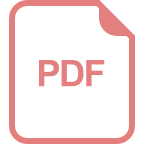
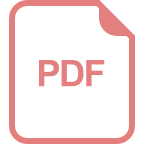
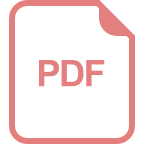













