.编写一个学生类,包括学号、姓名、年龄属性和一个构造方法,toString 方法。2) 主方法种键盘输入信息创建口个学生对象,将学生对象存放在集合中,按照年龄的高低排序输出. 要求:1) 如果年龄不是整数,则抛出输入错误异常;2)如果年龄小于了,拋出年龄低异常:如果年龄大于 35,则拋出年龄高异常;3) 如果姓名或学号为空,则抛出空异常。编写测试程序。编写自定义异常类,包括空异常类、年龄低异常类和年龄高 异常类。
时间: 2024-02-12 09:09:47 浏览: 66
以下是实现上述要求的代码:
```java
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.Scanner;
class Student {
private String studentID;
private String name;
private int age;
public Student(String studentID, String name, int age) {
this.studentID = studentID;
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Student{" +
"studentID='" + studentID + '\'' +
", name='" + name + '\'' +
", age=" + age +
'}';
}
public String getStudentID() {
return studentID;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
class AgeLowException extends Exception {
public AgeLowException() {
super("输入年龄过低");
}
}
class AgeHighException extends Exception {
public AgeHighException() {
super("输入年龄过高");
}
}
class InputErrorException extends Exception {
public InputErrorException() {
super("输入错误");
}
}
class NullException extends Exception {
public NullException() {
super("姓名或学号为空");
}
}
public class Main {
public static void main(String[] args) {
ArrayList<Student> studentList = new ArrayList<>();
Scanner scanner = new Scanner(System.in);
for (int i = 0; i < 5; i++) {
try {
System.out.println("请输入第" + (i + 1) + "个学生的信息:");
System.out.print("学号:");
String studentID = scanner.nextLine();
if (studentID.isEmpty()) {
throw new NullException();
}
System.out.print("姓名:");
String name = scanner.nextLine();
if (name.isEmpty()) {
throw new NullException();
}
System.out.print("年龄:");
int age = scanner.nextInt();
scanner.nextLine(); // 读取换行符
if (age < 18) {
throw new AgeLowException();
}
if (age > 35) {
throw new AgeHighException();
}
studentList.add(new Student(studentID, name, age));
} catch (AgeLowException | AgeHighException | InputErrorException | NullException e) {
System.out.println("输入错误:" + e.getMessage());
i--; // 重新输入
}
}
Collections.sort(studentList, Comparator.comparingInt(Student::getAge).reversed());
System.out.println(studentList);
}
}
```
在上面的代码中,我们首先定义了一个 `Student` 类,包括学号、姓名、年龄属性和一个构造方法,以及一个 `toString` 方法。然后,我们定义了三个自定义异常类:`AgeLowException` 表示输入年龄过低,`AgeHighException` 表示输入年龄过高,`NullException` 表示姓名或学号为空。在 `main` 方法中,我们使用一个 `for` 循环读取 5 个学生的信息,如果输入异常,则抛出相应的异常;否则创建一个 `Student` 对象,并将其存入一个 ArrayList 中。最后,我们使用 `Collections.sort` 方法按照年龄的高低排序输出。
阅读全文
相关推荐
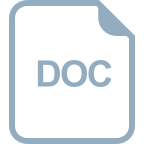
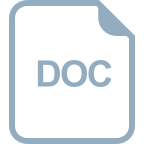
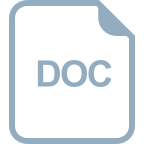




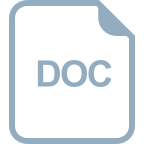
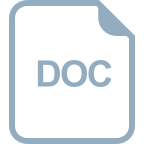
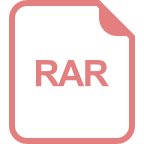
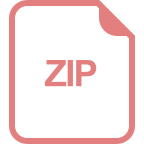
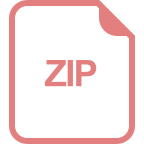
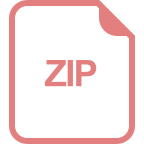
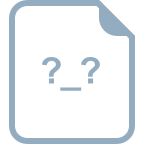