private void moveModel() { if (Input.touchCount == 0 || m_ARRaycastManager == null|| m_SelectedModel==null) return; var touch = Input.GetTouch(0); m_ARRaycastManager.Raycast(touch.position, m_Hits, TrackableType.Planes); if (m_Hits.Count > 0) { m_PlacementPose = m_Hits[0].pose; m_SelectedModel.transform.position = m_PlacementPose.position; } }
时间: 2023-06-26 12:09:38 浏览: 52
这段代码是在移动AR中的模型。首先,它检查是否有屏幕触摸事件、AR射线管理器和已选模型。如果没有这些条件,则不会执行任何操作。接着,它使用AR射线在平面上进行射线检测,并将检测结果存储在m_Hits列表中。如果射线检测到了平面,则将平面的位姿存储在m_PlacementPose中,并将已选模型的位置设置为平面的位置。这样就可以通过手指触摸屏幕来移动AR中的模型了。
相关问题
bot C# Expression<Func<T, bool>>如何实现传入Expression<Func<T, bool>> predicate 和 List<int> input,判断predicate是否为null,如果predicate是null输出形式为predicate = x => x.Id == input[0] || x.Id == input[1] || x.Id == input[2]的lambda表达式,如果predicate不是null输出形式为predicate.and(x => (x.Id == input[0] || x.Id == input[1] || x.Id == input[2]))的lambda表达式
您好!根据您的要求,我编写了以下代码:
```csharp
using System;
using System.Collections.Generic;
using System.Linq.Expressions;
class Program
{
static void Main(string[] args)
{
// 测试数据
List<int> input = new List<int> { 1, 2, 3 };
Expression<Func<Foo, bool>> predicate = null;
// 判断 predicate 是否为 null
if (predicate == null)
{
// 构造 lambda 表达式
predicate = x => x.Id == input[0] || x.Id == input[1] || x.Id == input[2];
}
else
{
// 构造 and 连接的 lambda 表达式
var parameter = predicate.Parameters[0];
var body = Expression.OrElse(
Expression.Equal(Expression.Property(parameter, "Id"), Expression.Constant(input[0])),
Expression.OrElse(
Expression.Equal(Expression.Property(parameter, "Id"), Expression.Constant(input[1])),
Expression.Equal(Expression.Property(parameter, "Id"), Expression.Constant(input[2]))
)
);
var lambda = Expression.Lambda<Func<Foo, bool>>(body, parameter);
predicate = predicate.And(lambda);
}
// 输出 lambda 表达式
Console.WriteLine(predicate);
}
}
class Foo
{
public int Id { get; set; }
// 其他成员...
}
public static class ExpressionExtensions
{
public static Expression<Func<T, bool>> And<T>(
this Expression<Func<T, bool>> expr1, Expression<Func<T, bool>> expr2)
{
var parameter = expr1.Parameters[0];
var body = Expression.AndAlso(expr1.Body, expr2.Body.ReplaceParameter(expr2.Parameters[0], parameter));
return Expression.Lambda<Func<T, bool>>(body, parameter);
}
private static Expression ReplaceParameter(this Expression expression, ParameterExpression source, ParameterExpression target)
{
return new ParameterReplacer { Source = source, Target = target }.Visit(expression);
}
private class ParameterReplacer : ExpressionVisitor
{
public ParameterExpression Source;
public ParameterExpression Target;
protected override Expression VisitParameter(ParameterExpression node)
{
return node == Source ? Target : base.VisitParameter(node);
}
}
}
```
其中,`Foo` 类是一个测试用的类,包含了一个整型属性 `Id`。`ExpressionExtensions` 类中的 `And` 方法用于将两个 `Expression<Func<T, bool>>` 类型的 lambda 表达式用 and 连接起来,`ReplaceParameter` 方法用于将一个 lambda 表达式中的参数替换为另一个参数。
在 `Main` 方法中,首先定义了一个 `input` 列表和一个 `predicate` lambda 表达式,然后根据 `predicate` 是否为 null 构造不同的 lambda 表达式,最后输出这个 lambda 表达式。
如果 `predicate` 为 null,则使用以下代码构造 lambda 表达式:
```csharp
predicate = x => x.Id == input[0] || x.Id == input[1] || x.Id == input[2];
```
如果 `predicate` 不为 null,则先使用以下代码构造一个 or 连接的 lambda 表达式:
```csharp
var parameter = predicate.Parameters[0];
var body = Expression.OrElse(
Expression.Equal(Expression.Property(parameter, "Id"), Expression.Constant(input[0])),
Expression.OrElse(
Expression.Equal(Expression.Property(parameter, "Id"), Expression.Constant(input[1])),
Expression.Equal(Expression.Property(parameter, "Id"), Expression.Constant(input[2]))
)
);
var lambda = Expression.Lambda<Func<Foo, bool>>(body, parameter);
```
然后使用 `And` 方法将这个 lambda 表达式和原来的 `predicate` 表达式连接起来:
```csharp
predicate = predicate.And(lambda);
```
最后输出这个 lambda 表达式即可:
```csharp
Console.WriteLine(predicate);
```
using System.Collections; using System.Collections.Generic; using UnityEngine; public class FollowTouch : MonoBehaviour { private Vector3 initialPosition; void Start() { initialPosition = transform.position; } void Update() { if (Input.touchCount > 0) { Touch touch = Input.GetTouch(0); Vector3 touchPosition = Camera.main.ScreenToWorldPoint(touch.position); touchPosition.z = 0f; transform.position = touchPosition; } float clampedX = Mathf.Clamp(transform.position.x, -5f, 5f); float clampedY = Mathf.Clamp(transform.position.y, -3f, 3f); transform.position = new Vector3(clampedX, clampedY, transform.position.z); } }上述代码运行后位置不对,拖动没有效果
根据你提供的代码,初步判断可能是因为物体的初始位置和拖动后的位置没有对齐导致的。你可以尝试在Update函数中添加一个判断条件,只有当触摸点触碰到物体时才继续执行拖动操作。修改后的代码如下:
```
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class FollowTouch : MonoBehaviour
{
private Vector3 initialPosition;
void Start()
{
initialPosition = transform.position;
}
void Update()
{
if (Input.touchCount > 0)
{
Touch touch = Input.GetTouch(0);
Vector3 touchPosition = Camera.main.ScreenToWorldPoint(touch.position);
touchPosition.z = 0f;
// 判断触摸点是否在物体上
Collider2D collider = Physics2D.OverlapPoint(touchPosition);
if (collider != null && collider.gameObject == gameObject)
{
transform.position = touchPosition;
}
}
float clampedX = Mathf.Clamp(transform.position.x, -5f, 5f);
float clampedY = Mathf.Clamp(transform.position.y, -3f, 3f);
transform.position = new Vector3(clampedX, clampedY, transform.position.z);
}
}
```
另外,你还可以在Unity编辑器中检查物体的碰撞器组件是否已经添加,并且是否正确设置了碰撞器的形状和大小。
相关推荐
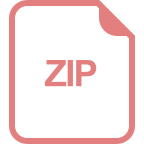
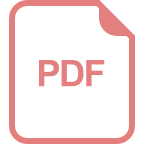
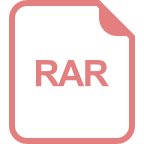













