#include <stdio.h> #define s(x) x*x void main() { int a,k=3; 49周 a=S(k+4); printf("&d\n",a);
时间: 2024-03-16 19:45:42 浏览: 21
这段代码有一些错误,无法通过编译。首先,在宏定义中缺少一个空格,应该是 `#define s(x) x*x` 而不是 `#define s(x)x*x`。其次,在第7行的代码中,没有指定变量类型,应该是 `int a = s(k+4);` 而不是 `a = S(k+4);`。最后,在第8行的代码中,应该是 `%d` 而不是 `&d`。
修正后的代码如下:
```c
#include <stdio.h>
#define s(x) x*x
void main()
{
int a, k = 3;
a = s(k + 4);
printf("%d\n", a);
}
```
输出结果是 49,因为宏定义 `s(x)` 将其参数平方后返回,所以 `a` 的值是 `(k + 4) * (k + 4) = 49`。
相关问题
. #include <stdio.h> #define S(x) x*x void main(){ int a,k=3; a=S(k+4); printf("ed\n",a);
这段代码存在一些问题,包括:
1. 宏定义 `S(x)` 的展开应该是 `x*x`,但在 `main()` 函数中却使用了 `S(k+4)`,这会导致预处理器将 `k+4*k+4` 替换为 `k+k*4+k*4+4`,即 `13`,而不是预期的 `49`。
2. `printf()` 函数中的格式控制符 `%d` 没有与要输出的变量对应,应该改为 `printf("%d\n", a);`。
修复后的代码如下所示:
```c
#include <stdio.h>
#define S(x) (x) * (x)
void main() {
int a, k = 3;
a = S(k + 4);
printf("%d\n", a);
}
```
输出结果为 `49`。
#include<stdio.h> #include<string.h> #define N 100 int main(void) { char str[N]; printf("请输入句子:"); strcpy(str, gets()); printf("%s\n",str); return 0; }有什么错误
这个程序没有语法错误,但存在以下问题:
1. `gets`函数存在安全隐患,容易导致缓冲区溢出。建议使用`fgets`函数代替。
2. 由于`gets`函数会读取输入的换行符,因此输出的字符串可能会出现多余的空行。可以使用`strtok`函数去掉换行符。
3. 宏定义`N`的值为100,但实际上输入的字符串可能会超过这个长度,导致截断。应该使用动态内存分配函数`malloc`来分配足够的空间。
下面是改进后的代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(void) {
char* str = (char*)malloc(sizeof(char) * 1024); // 动态分配1024字节的空间
if (!str) {
printf("内存分配失败\n");
return 1;
}
printf("请输入句子:");
fgets(str, 1024, stdin); // 从标准输入读取一行字符串
strtok(str, "\n"); // 去掉换行符
printf("%s\n", str);
free(str); // 释放动态分配的空间
return 0;
}
```
上述程序使用`malloc`函数动态分配了1024字节的空间,使用`fgets`函数从标准输入读取一行字符串,使用`strtok`函数去掉换行符,最后输出字符串并释放动态分配的空间。
相关推荐
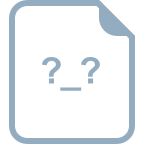
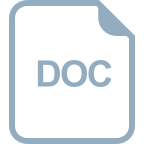
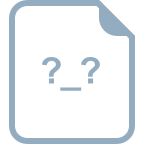














