用Spill Tree选取与一个数据近邻的数据的索引的Python代码
时间: 2024-03-12 07:46:58 浏览: 165
Spill Tree是一种基于kd-tree的近似最近邻搜索算法,它在搜索效率和精度之间取得了一个平衡。虽然scikit-learn库中没有Spill Tree算法的实现,但是可以使用pynndescent库来实现。
以下是使用pynndescent库实现Spill Tree选取数据近邻索引的Python代码示例:
```python
from pynndescent import NNDescent
# 构造数据集
X = [[0], [1], [2], [3]]
# 构建Spill Tree
tree = NNDescent(X, metric='euclidean')
# 选择查询点
query_point = [[1.5]]
# 选取最近邻
dist, ind = tree.query(query_point, k=1)
# 打印选取的最近邻索引
print(ind)
```
代码中,我们先构造了一个二维数据集X,然后使用NNDescent算法构建了一个Spill Tree。接着,我们选择一个查询点query_point,并使用tree.query方法选取了与query_point最近的数据点的索引。最后,我们打印出了选取的最近邻索引ind。需要注意的是,使用pynndescent库需要先安装该库,安装方法可参考pynndescent的官方文档。
相关问题
从https://www.kaggle.com/datasets上爬取Oil Spill Classifications数据的python代码
### 回答1:
首先,需要安装爬虫库beautifulsoup和requests,可以使用pip进行安装。
代码如下:
```python
import requests
from bs4 import BeautifulSoup
# 爬取网页
url = "https://www.kaggle.com/datasets"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 查找数据集名称为"Oil Spill Classifications"的链接
link = soup.find("a", text="Oil Spill Classifications")["href"]
# 访问数据集下载链接
url = "https://www.kaggle.com" + link + "/download"
response = requests.get(url)
# 保存数据集
with open("oil_spill_classifications.csv", "wb") as f:
f.write(response.content)
```
这是一个爬取kaggle上oil spill classifications 数据集的简单示例,在实际中需要考虑防止爬虫被封禁等问题。
### 回答2:
以下是一个用Python爬取Kaggle上Oil Spill Classifications数据的示例代码:
```python
import requests
# 定义要爬取的数据集的URL
url = "https://www.kaggle.com/datasets"
# 发送HTTP GET请求获取网页内容
response = requests.get(url)
if response.status_code == 200:
# 解析网页内容,提取数据集的下载链接
dataset_links = []
# 使用合适的库进行网页解析
# 这里以BeautifulSoup为例
from bs4 import BeautifulSoup
soup = BeautifulSoup(response.text, "html.parser")
dataset_divs = soup.find_all("div", class_="dataset-list-item")
for dataset_div in dataset_divs:
# 可按需要进一步筛选数据集
link = dataset_div.find("a", class_="dataset-item-title").get("href")
dataset_links.append(link)
# 下载数据集
for link in dataset_links:
# 构造具体数据集的URL
dataset_url = "https://www.kaggle.com" + link
# 发送HTTP GET请求下载数据集
dataset_response = requests.get(dataset_url)
if dataset_response.status_code == 200:
# 保存数据集到本地文件
file_name = link.split("/")[-1] + ".csv"
with open(file_name, "wb") as file:
file.write(dataset_response.content)
print("数据集下载完成:", file_name)
else:
print("数据集下载失败:", dataset_url)
else:
print("获取网页内容失败:", url)
```
请注意,爬取Kaggle数据集需要先登录,并在代码中提供相应的身份验证。以上代码只是示例,具体的身份验证方式可能需要根据实际情况进行调整。同时,代码还需要根据实际网页结构进行适当的修改,以确保正确解析并找到目标数据集的下载链接。
### 回答3:
以下是从https://www.kaggle.com/datasets上爬取Oil Spill Classifications数据的Python代码:
```python
import requests
from bs4 import BeautifulSoup
# 定义目标网址
url = "https://www.kaggle.com/datasets"
# 发送HTTP请求并获取网页内容
response = requests.get(url)
# 使用BeautifulSoup解析网页内容
soup = BeautifulSoup(response.text, "html.parser")
# 查找包含Oil Spill Classifications数据的相关链接
dataset_links = soup.find_all("a", href=True, text="Oil Spill Classifications")
# 提取第一个相关链接
oil_spill_dataset_link = dataset_links[0]["href"]
# 发送HTTP请求并获取Oil Spill Classifications数据集页面内容
oil_spill_response = requests.get(oil_spill_dataset_link)
# 使用BeautifulSoup解析数据集页面内容
oil_spill_soup = BeautifulSoup(oil_spill_response.text, "html.parser")
# 查找下载按钮链接
download_button = oil_spill_soup.find("a", class_="site-click", text="Download")
# 提取下载链接
download_link = download_button["href"]
# 发送HTTP请求并下载文件
file_response = requests.get(download_link)
# 保存数据文件
with open("oil_spill_classification_data.csv", "wb") as file:
file.write(file_response.content)
print("数据已成功下载并保存为oil_spill_classification_data.csv文件。")
```
使用这段代码,你将能够从https://www.kaggle.com/datasets网站上爬取Oil Spill Classifications数据,并将数据保存为名为oil_spill_classification_data.csv的文件。
给出用python爬取https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection数据的代码
### 回答1:
下面是一个使用 Python 爬取 https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection 的代码示例:
```
import requests
url = 'https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection'
response = requests.get(url)
with open('oil-spill-detection.csv', 'wb') as f:
f.write(response.content)
```
这段代码使用了 Python 中 requests 库来发送 GET 请求,并将返回的数据保存在当前目录下的 oil-spill-detection.csv 文件中。
需要注意的是,如果这个数据集需要登录才能下载的话,需要额外的操作来登录才能爬取到数据。
### 回答2:
以下是使用Python爬取https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection数据的示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 请求页面
url = 'https://www.kaggle.com/datasets/sudhanshu2198/oil-spill-detection'
response = requests.get(url)
# 解析页面内容
soup = BeautifulSoup(response.text, 'html.parser')
# 查找下载链接
download_link = soup.find('div', class_='download-button-container') \
.find('a', class_='site-button')['href']
# 下载数据文件
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
data_response = requests.get(download_link, headers=headers)
# 保存数据文件
with open('oil_spill_detection.zip', 'wb') as file:
file.write(data_response.content)
print('数据爬取完成并保存成功!')
```
这段代码首先使用`requests`库发送HTTP请求,获取网页的HTML内容。然后,使用` BeautifulSoup`库解析HTML内容,查找下载链接的位置。接着,使用`requests`库再次发送HTTP请求,下载数据文件。最后,将下载的数据文件保存到本地。
阅读全文
相关推荐
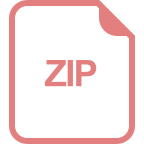
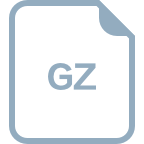
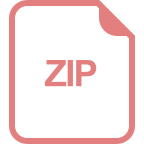








