写一个C语言程序将合法C源程序每行前加上行号并删除其所有注释
时间: 2023-06-08 19:03:29 浏览: 176
当然可以,这是一个常见的需求。下面是一个简单的实现:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LINE_LEN 1024
int main(int argc, char **argv) {
if (argc != 2) {
printf("Usage: addline input_file\n");
return 1;
}
FILE *input_file = fopen(argv[1], "r");
if (input_file == NULL) {
printf("Cannot open file %s\n", argv[1]);
return 2;
}
char *line_buf = malloc(sizeof(char) * MAX_LINE_LEN);
if (line_buf == NULL) {
printf("Cannot allocate memory for line buffer\n");
return 3;
}
char *comment_start;
int line_num = 1;
while (fgets(line_buf, MAX_LINE_LEN, input_file) != NULL) {
// Remove trailing newline character
line_buf[strcspn(line_buf, "\r\n")] = '\0';
// Find start of comment and remove it
comment_start = strstr(line_buf, "//");
if (comment_start != NULL) {
*comment_start = '\0';
}
// Print line with line number
printf("%d: %s\n", line_num, line_buf);
line_num++;
}
free(line_buf);
fclose(input_file);
return 0;
}
```
这个程序读入一个 C 语言源文件,输出一个带行号的版本,并且删除每行的注释。几点说明:
- 程序使用了 fgets 函数逐行读入源程序。MAX_LINE_LEN 宏定义了一行最多能包含的字符数。
- 当遇到注释时,程序通过 strstr 函数找到注释的位置,然后将它后面的部分截掉。这里只考虑了单行注释,如果源程序中还有多行注释、字符常量或字符串,还需要对其进行识别和处理。
- 最后程序使用 printf 输出带行号的源程序。
阅读全文
相关推荐
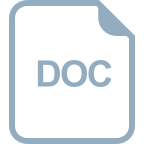
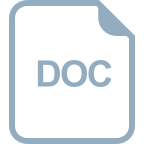
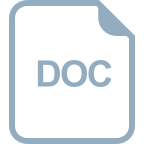















