利用消息队列实现两个进程之间的通信。 要求: (1)编写两个程序:读消息队列程序(进程A)和写消息队列程序(进程B)。 (2)通过消息队列实现两个进程相互循环通信,即通过同一个消息队列进程A给进程B发送消息,然后进程B给进程A发送消息,往复循环。 (3)写入消息队列的内容从键盘上获得。 (4)将接收到的消息内容输出到屏幕上。 提醒:要想实现进程A和进程B 通过同一个消息队列相互通信,方法是可以设置不同的消息类型,通过类型去读消息。
时间: 2024-03-02 13:52:21 浏览: 172
好的,这是一个非常典型的进程间通信问题,我来给您详细地讲解一下。
首先,我们需要了解一下什么是消息队列。消息队列是一种进程间通信机制,它允许一个或多个进程向队列中添加消息,而另外的进程则可以从队列中取出消息。在 Linux 系统中,消息队列是一种 IPC(Inter-Process Communication,进程间通信)的方式,它通常被用来解决生产者-消费者问题,或者是进程间的信号量同步问题。
下面,我来介绍一下如何使用消息队列实现两个进程之间的通信。
首先,我们需要定义一个消息结构体,它包含了消息的类型和内容。在这里,我们定义了两个类型,分别为 1 和 2。
```
struct msgbuf {
long mtype; // 消息类型
char mtext[1024]; // 消息内容
};
```
接下来,我们需要编写两个程序:读消息队列程序(进程A)和写消息队列程序(进程B)。
进程A的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
int main()
{
key_t key;
int msqid;
struct msgbuf rcvbuf;
// 生成 key
key = ftok(".", 'a');
if (key == -1) {
perror("ftok");
exit(1);
}
// 创建消息队列
msqid = msgget(key, 0666 | IPC_CREAT);
if (msqid == -1) {
perror("msgget");
exit(1);
}
while (1) {
// 接收消息类型为 2 的消息
if (msgrcv(msqid, &rcvbuf, sizeof(rcvbuf.mtext), 2, 0) == -1) {
perror("msgrcv");
exit(1);
}
// 输出接收到的消息
printf("Received message: %s\n", rcvbuf.mtext);
// 发送消息类型为 1 的消息
printf("Send message: ");
fgets(rcvbuf.mtext, sizeof(rcvbuf.mtext), stdin);
rcvbuf.mtype = 1;
if (msgsnd(msqid, &rcvbuf, strlen(rcvbuf.mtext) + 1, 0) == -1) {
perror("msgsnd");
exit(1);
}
}
return 0;
}
```
进程B的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/msg.h>
int main()
{
key_t key;
int msqid;
struct msgbuf sendbuf;
// 生成 key
key = ftok(".", 'a');
if (key == -1) {
perror("ftok");
exit(1);
}
// 获取消息队列 ID
msqid = msgget(key, 0666);
if (msqid == -1) {
perror("msgget");
exit(1);
}
while (1) {
// 发送消息类型为 2 的消息
printf("Send message: ");
fgets(sendbuf.mtext, sizeof(sendbuf.mtext), stdin);
sendbuf.mtype = 2;
if (msgsnd(msqid, &sendbuf, strlen(sendbuf.mtext) + 1, 0) == -1) {
perror("msgsnd");
exit(1);
}
// 接收消息类型为 1 的消息
if (msgrcv(msqid, &sendbuf, sizeof(sendbuf.mtext), 1, 0) == -1) {
perror("msgrcv");
exit(1);
}
// 输出接收到的消息
printf("Received message: %s\n", sendbuf.mtext);
}
return 0;
}
```
在这里,我们使用了 ftok 函数生成唯一的 key,然后使用 msgget 函数创建或获取消息队列 ID。在进程A中,我们使用 msgrcv 函数接收类型为 2 的消息,然后使用 msgsnd 函数发送类型为 1 的消息。在进程B中,我们先使用 msgsnd 函数发送类型为 2 的消息,然后使用 msgrcv 函数接收类型为 1 的消息。
最后,我们编译并运行这两个程序,它们就可以相互循环通信了。
阅读全文
相关推荐
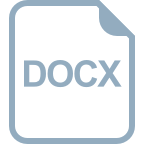


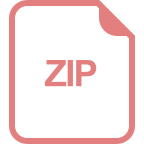
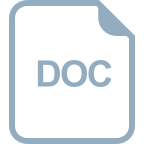
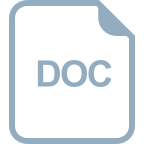
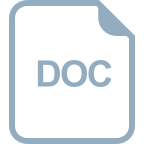
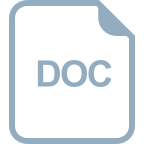
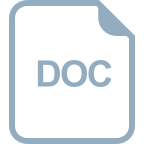
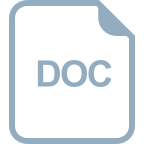
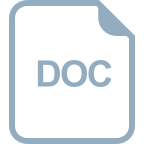





