巴特沃斯滤波器c语言源码
时间: 2023-12-05 12:05:29 浏览: 63
巴特沃斯滤波器是一种常用的数字滤波器,可以用于滤波信号中的高频噪声。以下是一个基于C语言的巴特沃斯滤波器的源码:
```c
#include <stdio.h>
#include <math.h>
#define PI 3.14159265358979323846
void butterworth_filter(double *input, double *output, int n, double cutoff, double sample_rate);
int main() {
double input[] = {1.0, 2.0, 3.0, 4.0, 5.0, 4.0, 3.0, 2.0, 1.0};
double output[9];
double cutoff = 5.0;
double sample_rate = 20.0;
int n = 3;
butterworth_filter(input, output, n, cutoff, sample_rate);
int i;
for (i = 0; i < 9; i++) {
printf("%f\n", output[i]);
}
return 0;
}
void butterworth_filter(double *input, double *output, int n, double cutoff, double sample_rate) {
double w0 = 2 * PI * cutoff / sample_rate;
double c = cos(w0);
double s = sin(w0);
double alpha = s / (2 * pow(M_E, log(2.0) / 2.0 / n));
double b[3], a[3];
b[0] = 1.0 / (1.0 + 2.0 * alpha * c + pow(alpha, 2.0));
b[1] = 2 * b[0];
b[2] = b[0];
a[0] = 1.0;
a[1] = 2.0 * (pow(alpha, 2.0) - 1.0) * b[0];
a[2] = (1.0 - 2.0 * alpha * c + pow(alpha, 2.0)) * b[0];
double x[3] = {0.0, 0.0, 0.0};
double y[3] = {0.0, 0.0, 0.0};
int i;
for (i = 0; i < 9; i++) {
x[0] = x[1];
x[1] = x[2];
x[2] = input[i];
y[0] = y[1];
y[1] = y[2];
y[2] = b[0] * x[2] + b[1] * x[1] + b[2] * x[0] - a[1] * y[1] - a[2] * y[0];
output[i] = y[2];
}
}
```
在上面的代码中,我们定义了一个 `butterworth_filter` 函数来实现巴特沃斯滤波器。该函数接受一个输入数组 `input`,一个输出数组 `output`,一个整数 `n`,一个截止频率 `cutoff`,和一个采样率 `sample_rate`。在函数中,我们首先计算出巴特沃斯滤波器的各个参数,然后使用一个循环来遍历输入数组,并根据公式计算出输出数组中的每个元素。
在主函数中,我们定义了一个输入数组和一些参数,然后调用 `butterworth_filter` 函数来计算输出数组,并将输出数组的值打印出来。
相关推荐
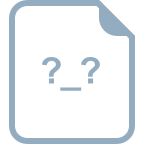














