如何使用pthread_create
时间: 2023-09-08 17:09:33 浏览: 80
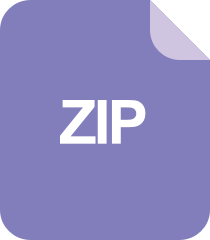
myweb.zip_网页服务器
pthread_create函数是POSIX线程库中的一个函数,用于创建一个新的线程。其函数原型如下:
```c
#include <pthread.h>
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine) (void *), void *arg);
```
其中,参数含义如下:
- thread: 指向线程标识符的指针,由该函数返回。
- attr: 指向线程属性的指针,可以用来设置线程的一些属性,如栈大小等,如果不需要设置属性,可以传入NULL。
- start_routine: 指向线程函数的指针,该函数的返回值和参数都是void *类型,即可以接收任何类型的参数,并返回任何类型的值。
- arg: 传递给线程函数的参数。
下面是一个简单的例子,演示如何使用pthread_create创建一个新的线程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
void *print_hello(void *arg) {
printf("Hello, world!\n");
pthread_exit(NULL);
}
int main() {
pthread_t tid;
int ret = pthread_create(&tid, NULL, print_hello, NULL);
if (ret != 0) {
printf("Error: pthread_create failed.\n");
exit(EXIT_FAILURE);
}
pthread_join(tid, NULL);
return 0;
}
```
在上面的例子中,我们定义了一个函数print_hello,它的作用是打印“Hello, world!”。然后我们在主函数中调用pthread_create函数创建一个新的线程,并将print_hello函数作为线程函数传递给它。在pthread_create函数成功创建线程后,我们调用pthread_join函数等待线程结束,并在主线程中打印出“Hello, world!”。
需要注意的是,在使用pthread_create创建新线程时,如果传递给线程函数的参数是引用类型,需要注意线程函数结束后所引用的内存是否已经被释放。如果被释放,可能会导致程序崩溃或产生不可预期的结果。
阅读全文
相关推荐
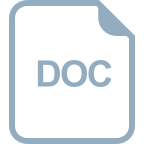
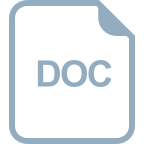















