如何对reducer进行排序
时间: 2023-07-26 15:49:23 浏览: 100
在Hive中,可以使用 `SORT BY` 关键字对 reducer 的输出进行排序。 `SORT BY` 可以在 `SELECT` 语句中使用,例如:
```
SELECT col1, col2, col3
FROM my_table
SORT BY col1 ASC, col2 DESC;
```
在上面的例子中,`my_table` 的数据会被分配到 reducer 中进行处理,并且在 reducer 输出结果之前,数据会按照 `col1` 升序排序,然后按照 `col2` 降序排序。
需要注意的是,使用 `SORT BY` 时,数据必须被分配到 reducer 中,否则不能进行排序。因此,如果你想对所有的结果进行排序,应该使用 `ORDER BY` 关键字。
另外,由于 `SORT BY` 只对 reducer 的输出进行排序,因此它的执行速度通常比 `ORDER BY` 更快,特别是在处理大量数据时。
相关问题
MapReduce使用快速排序算法实现对文件里面数字进行排序的java代码
下面是使用MapReduce实现对文件里面数字进行排序的Java代码,其中使用了快速排序算法:
```java
import java.io.IOException;
import java.util.*;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.*;
import org.apache.hadoop.mapreduce.*;
public class SortNumbers {
public static class Map extends Mapper<LongWritable, Text, IntWritable, NullWritable> {
private IntWritable num = new IntWritable();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String line = value.toString();
StringTokenizer tokenizer = new StringTokenizer(line);
while (tokenizer.hasMoreTokens()) {
num.set(Integer.parseInt(tokenizer.nextToken()));
context.write(num, NullWritable.get());
}
}
}
public static class Reduce extends Reducer<IntWritable, NullWritable, IntWritable, NullWritable> {
public void reduce(IntWritable key, Iterable<NullWritable> values, Context context) throws IOException, InterruptedException {
context.write(key, NullWritable.get());
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "sort numbers");
job.setJarByClass(SortNumbers.class);
job.setMapperClass(Map.class);
job.setReducerClass(Reduce.class);
job.setOutputKeyClass(IntWritable.class);
job.setOutputValueClass(NullWritable.class);
job.setNumReduceTasks(1); // 设置Reduce任务数为1
// 设置输入和输出文件路径
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
在这段代码中,Map任务将输入文件按照空格分隔符进行拆分,将每个数字作为key值输出,value值为NullWritable。Reduce任务将输入的key值进行全局排序,并输出结果。最终结果会按照升序排列的顺序输出到输出文件中。
编写 MapReduce 程序,对文件中的数据进行排序处理
下面是一个简单的 MapReduce 排序程序,可以对输入文件中的数据进行排序处理:
Map 阶段:
```python
def mapper(_, line):
# 将每一行数据拆分成键值对,并将键值对的 key 设置为需要排序的值
value = int(line.strip())
yield value, None
```
Reduce 阶段:
```python
def reducer(key, _):
# 直接输出 key 即为排序结果
yield key, None
```
在 Hadoop 中运行该程序时,需要使用以下命令:
```
hadoop jar /path/to/hadoop-streaming.jar \
-input /path/to/input/file \
-output /path/to/output/directory \
-mapper "python /path/to/mapper.py" \
-reducer "python /path/to/reducer.py" \
-numReduceTasks 1 \
-file /path/to/mapper.py \
-file /path/to/reducer.py
```
其中 `-numReduceTasks` 参数可以指定 Reduce 任务的数量,如果只有一个 Reduce 任务,则输出结果即为排序结果。
阅读全文
相关推荐
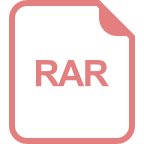
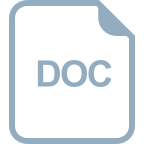
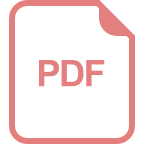
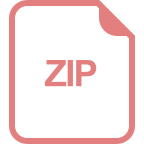
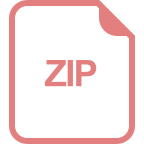
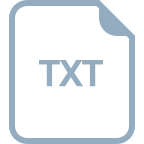
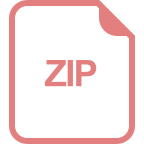
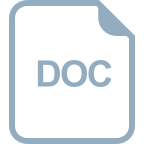
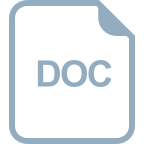
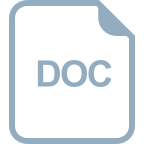
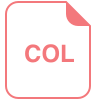
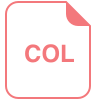
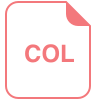
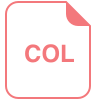



