编写main函数测试用带头节点的单链表来实现的线性表,并检测其线性表的基本功能
时间: 2024-06-03 17:08:36 浏览: 158
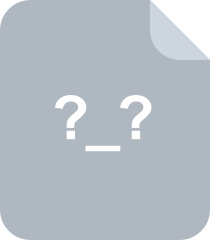
将带头结点的单链表实现线性表的功能

#include <iostream>
using namespace std;
// 定义链表节点结构体
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 定义带头节点的单链表类
class LinkedList {
private:
ListNode* head;
public:
LinkedList() {
head = new ListNode(0);
}
// 在链表末尾添加节点
void add(int val) {
ListNode* cur = head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = new ListNode(val);
}
// 在链表指定位置插入节点
void insert(int index, int val) {
int count = 0;
ListNode* cur = head;
while (cur != NULL && count != index) {
cur = cur->next;
count++;
}
if (cur != NULL) {
ListNode* newNode = new ListNode(val);
newNode->next = cur->next;
cur->next = newNode;
}
}
// 删除链表指定位置的节点
void remove(int index) {
int count = 0;
ListNode* cur = head;
while (cur != NULL && count != index) {
cur = cur->next;
count++;
}
if (cur != NULL && cur->next != NULL) {
ListNode* temp = cur->next;
cur->next = temp->next;
delete temp;
}
}
// 获取链表指定位置的节点的值
int get(int index) {
int count = 0;
ListNode* cur = head->next;
while (cur != NULL && count != index) {
cur = cur->next;
count++;
}
if (cur != NULL) {
return cur->val;
} else {
return -1;
}
}
// 修改链表指定位置的节点的值
void set(int index, int val) {
int count = 0;
ListNode* cur = head->next;
while (cur != NULL && count != index) {
cur = cur->next;
count++;
}
if (cur != NULL) {
cur->val = val;
}
}
// 获取链表长度
int size() {
int count = 0;
ListNode* cur = head->next;
while (cur != NULL) {
cur = cur->next;
count++;
}
return count;
}
// 打印链表
void print() {
ListNode* cur = head->next;
while (cur != NULL) {
cout << cur->val << " ";
cur = cur->next;
}
cout << endl;
}
};
int main() {
LinkedList list;
list.add(1);
list.add(2);
list.add(3);
list.print(); // 打印:1 2 3
list.insert(1, 4);
list.print(); // 打印:1 4 2 3
list.remove(2);
list.print(); // 打印:1 4 3
cout << list.get(1) << endl; // 打印:4
list.set(2, 5);
list.print(); // 打印:1 4 5
cout << list.size() << endl; // 打印:3
return 0;
}
阅读全文
相关推荐















