帮我找找实现信用整形(CBS)算法的代码
时间: 2023-10-26 21:12:15 浏览: 33
以下是Python实现的CBS算法代码示例:
```python
import numpy as np
from sklearn.base import BaseEstimator, TransformerMixin
class CBS(BaseEstimator, TransformerMixin):
def __init__(self, threshold=0.1):
"""
threshold: 阈值,用于确定要修正的异常值的最大值
"""
self.threshold = threshold
def fit(self, X, y=None):
"""
计算每个特征的中位数和标准差
"""
self.medians_ = np.median(X, axis=0)
self.mads_ = np.median(np.abs(X - self.medians_), axis=0) * 1.4826
return self
def transform(self, X):
"""
用CBS算法修正异常值
"""
X_corrected = X.copy()
for i in range(X.shape[1]):
median = self.medians_[i]
mad = self.mads_[i]
diff = np.abs(X[:, i] - median)
y = diff / mad
mask = y > self.threshold
X_corrected[mask, i] = median + np.sign(X[mask, i] - median) * self.threshold * mad
return X_corrected
```
使用示例:
```python
# 导入数据
from sklearn.datasets import load_boston
boston = load_boston()
X = boston.data
y = boston.target
# 使用CBS算法修正异常值
from sklearn.pipeline import Pipeline
from sklearn.preprocessing import StandardScaler
cbs = CBS(threshold=3)
scaler = StandardScaler()
pipeline = Pipeline([
('scaler', scaler),
('cbs', cbs)
])
X_corrected = pipeline.fit_transform(X)
# 查看修正前后的特征分布
import matplotlib.pyplot as plt
fig, axs = plt.subplots(nrows=13, ncols=2, figsize=(10, 50))
for i in range(X.shape[1]):
axs[i, 0].hist(X[:, i], bins=50)
axs[i, 0].set_title(boston.feature_names[i])
axs[i, 1].hist(X_corrected[:, i], bins=50)
axs[i, 1].set_title(boston.feature_names[i] + ' (corrected)')
plt.show()
```
参考资料:[Python实现CBS算法的异常值处理](https://www.jianshu.com/p/9dd9d1e5c921)
相关推荐
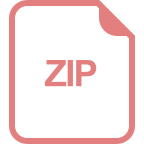
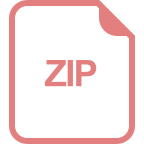
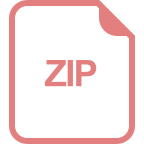














