python摄像头一直打开,知道其轮廓周长大于1/2时,开始采集从这一时刻开始到3秒后结束或者前100帧的所有图像左极值点,并将所有左极值点垂直像素进行排序,求出最大值和最小值之差
时间: 2023-05-29 17:04:17 浏览: 43
这里给出一个可能的实现:
```python
import cv2
import time
# 打开摄像头
cap = cv2.VideoCapture(0)
# 初始化相关变量
start_time = None
left_points = []
max_y = -1
min_y = 100000
while True:
# 读取一帧图像
ret, frame = cap.read()
# 转为灰度图并进行阈值化处理
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
_, thresh = cv2.threshold(gray, 100, 255, cv2.THRESH_BINARY)
# 计算轮廓并找到最长的轮廓
contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
max_contour = None
max_contour_length = -1
for contour in contours:
length = cv2.arcLength(contour, True)
if length > max_contour_length:
max_contour = contour
max_contour_length = length
# 如果轮廓周长大于1/2,则开始采集左极值点
if max_contour_length > gray.shape[1] // 2:
if start_time is None:
start_time = time.time()
if len(left_points) < 100 and time.time() - start_time < 3:
leftmost = tuple(max_contour[max_contour[:,:,0].argmin()][0])
left_points.append(leftmost[1])
if leftmost[1] > max_y:
max_y = leftmost[1]
if leftmost[1] < min_y:
min_y = leftmost[1]
else:
break
# 显示当前帧
cv2.imshow('frame', frame)
# 按下q键退出
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放摄像头并关闭所有窗口
cap.release()
cv2.destroyAllWindows()
# 对左极值点垂直像素进行排序并求出最大值和最小值之差
left_points.sort()
diff = left_points[-1] - left_points[0]
print('max_y:', max_y)
print('min_y:', min_y)
print('diff:', diff)
```
注:上述代码中的阈值、轮廓查找方式等参数需要根据具体情况进行调整。
相关推荐
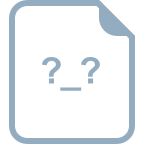















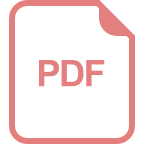