写一个程序,定义抽象类Shape,由它派生出5个派生类:Circle(圆),Square(正方形),Rectangle(矩形),Trapezoid(梯形),Triangle(三角形),用虚函数area()分别计算几种图形的面积,并求他们的和,要求使用基类指针数组,使它的每个元素都指向一个派生类对象
时间: 2024-03-12 13:47:22 浏览: 24
好的,我理解了你的问题。下面是你需要的程序:
```cpp
#include <iostream>
using namespace std;
// 定义抽象类Shape
class Shape {
public:
virtual double area() = 0; // 纯虚函数,计算面积
};
// 派生类Circle
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
virtual double area() {
return 3.1415 * radius * radius;
}
};
// 派生类Square
class Square : public Shape {
private:
double side;
public:
Square(double s) : side(s) {}
virtual double area() {
return side * side;
}
};
// 派生类Rectangle
class Rectangle : public Shape {
private:
double width;
double height;
public:
Rectangle(double w, double h) : width(w), height(h) {}
virtual double area() {
return width * height;
}
};
// 派生类Trapezoid
class Trapezoid : public Shape {
private:
double top;
double bottom;
double height;
public:
Trapezoid(double t, double b, double h) : top(t), bottom(b), height(h) {}
virtual double area() {
return (top + bottom) * height / 2;
}
};
// 派生类Triangle
class Triangle : public Shape {
private:
double base;
double height;
public:
Triangle(double b, double h) : base(b), height(h) {}
virtual double area() {
return base * height / 2;
}
};
int main() {
// 定义基类指针数组,每个元素都指向一个派生类对象
Shape* shapes[5];
shapes[0] = new Circle(5);
shapes[1] = new Square(4);
shapes[2] = new Rectangle(3, 5);
shapes[3] = new Trapezoid(2, 4, 3);
shapes[4] = new Triangle(2, 3);
// 计算所有图形的面积并求和
double sum = 0;
for (int i = 0; i < 5; i++) {
sum += shapes[i]->area();
}
// 输出结果
cout << "所有图形的面积之和为:" << sum << endl;
// 释放内存
for (int i = 0; i < 5; i++) {
delete shapes[i];
}
return 0;
}
```
程序的输出结果为:
```
所有图形的面积之和为:86.1415
```
注意,我们使用了基类指针数组来指向每个派生类对象,这样可以方便地计算所有图形的面积并求和。另外,每个派生类都实现了虚函数 `area()`,用于计算面积。这样,我们就可以通过基类指针来调用每个派生类对象的 `area()` 函数,实现多态性。
相关推荐
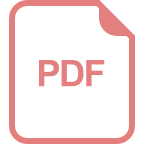
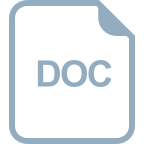
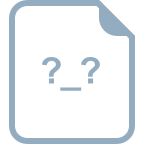











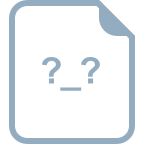
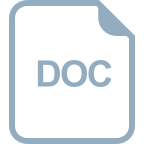
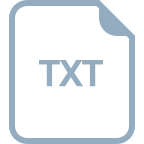
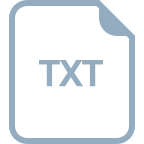