module ram_test; // Inputs reg clka; reg [0:0] wea; reg [5:0] addra; reg [31:0] dina; // Outputs wire [31:0] douta; // Instantiate the Unit Under Test (UUT) ram uut ( .clka(clka), .wea(wea), .addra(addra), .dina(dina), .douta(douta) ); reg[63:0]data[0:31]; initial begin // Initialize Inputs clka = 0; wea = 0; addra = 0; dina = 0; end // Wait 100 ns for global reset to finish // 时钟信号 initial begin clka = 0; forever #10 clka = ~clka; end // 写入数据 initial begin wea = 1; addra = 0; repeat (64*4) begin // 从文件中读取数据 $readmemh("data_file.txt", data); // 将数据写入存储器 dina = data[addra % 64]; #10; addra = addra + 1; end wea = 0; end // 读取数据 initial begin addra = 0; repeat (16) begin #10; $display("Data at address %0d: %h", addra, douta); addra = addra + 1; end end endmodulemodule ram_test; // Inputs reg clka; reg [0:0] wea; reg [5:0] addra; reg [31:0] dina; // Outputs wire [31:0] douta; // Instantiate the Unit Under Test (UUT) ram uut ( .clka(clka), .wea(wea), .addra(addra), .dina(dina), .douta(douta) ); reg[63:0]data[0:31]; initial begin // Initialize Inputs clka = 0; wea = 0; addra = 0; dina = 0; end // Wait 100 ns for global reset to finish // 时钟信号 initial begin clka = 0; forever #10 clka = ~clka; end // 写入数据 initial begin wea = 1; addra = 0; repeat (64*4) begin // 从文件中读取数据 $readmemh("data_file.txt", data); // 将数据写入存储器 dina = data[addra % 64]; #10; addra = addra + 1; end wea = 0; end // 读取数据 initial begin addra = 0; repeat (16) begin #10; $display("Data at address %0d: %h", addra, douta); addra = addra + 1; end end endmodule
时间: 2024-04-28 15:20:09 浏览: 111
这段代码是一个简单的 Verilog 代码,用于测试 RAM 存储器模块。代码中包含了 RAM 模块的输入和输出信号,以及存储器的初始化、写入和读取操作。
在初始化过程中,输入信号被初始化为 0,然后通过时钟信号进行重置。然后,通过 `$readmemh` 函数从文件中读取数据,并将数据写入存储器。最后,通过 `$display` 函数输出存储器中的数据。
需要注意的是,代码中使用了 Verilog 的一些语法,例如 `initial` 和 `repeat` 关键字,以及 `$readmemh` 和 `$display` 系统函数。如果您不熟悉 Verilog 语言,建议先学习一下 Verilog 的基本语法。
相关问题
module ram_test; // Inputs reg clka; reg [0:0] wea; reg [5:0] addra; reg [31:0] dina; // Outputs wire [31:0] douta; // Instantiate the Unit Under Test (UUT) ram uut ( .clka(clka), .wea(wea), .addra(addra), .dina(dina), .douta(douta) );给我一个测试文件
以下是一个简单的测试文件,用于测试上述 `ram_test` 模块:
```
`timescale 1ns / 1ps
module tb_ram;
// Inputs
reg clka;
reg [0:0] wea;
reg [5:0] addra;
reg [31:0] dina;
// Outputs
wire [31:0] douta;
// Instantiate the Unit Under Test (UUT)
ram_test uut (
.clka(clka),
.wea(wea),
.addra(addra),
.dina(dina),
.douta(douta)
);
initial begin
// Initialize Inputs
clka = 0;
wea = 0;
addra = 0;
dina = 0;
// Wait 100 ns for global reset to finish
#100;
// Write data to memory
wea = 1;
addra = 0;
repeat (64) begin
dina = $random;
#10;
addra = addra + 1;
end
wea = 0;
// Read data from memory
addra = 0;
repeat (16) begin
#10;
$display("Data at address %0d: %h", addra, douta);
addra = addra + 1;
end
$finish;
end
endmodule
```
这个测试文件实例化了上述 `ram_test` 模块,并对其进行测试。在初始块中,先对输入端口进行了初始化。然后使用 `$random` 系统函数生成随机数据,写入存储器。接着,从存储器中读取数据并将其输出。整个过程使用时钟信号 `clka` 来同步存储器的读写操作。最后使用 `$finish` 系统任务结束仿真。
`timescale 1ns / 1ps module test_tb; // Inputs reg clk; reg reset; // Outputs wire [7:0] led; // Instantiate the Unit Under Test (UUT) led uut ( .clk(clk), .reset(reset), .led(led) ); always #10 clk =~clk; initial begin // Initialize Inputs clk = 0; reset = 1; // Wait 100 ns for global reset to finish #100; reset = 0; // Add stimulus here end endmodule 解释这段代码,并每一行都添加注释
这段代码是一个 Verilog 语言的测试模块,用于测试一个名为 "led" 的模块。以下是每一行的解释:
```
`timescale 1ns / 1ps // 定义时间单位为1纳秒/1皮秒
module test_tb; // 定义模块名为 test_tb
// Inputs
reg clk; // 定义输入信号 clk 为寄存器类型
reg reset; // 定义输入信号 reset 为寄存器类型
// Outputs
wire [7:0] led; // 定义输出信号 led 为线网类型,宽度为8位
// Instantiate the Unit Under Test (UUT)
led uut ( // 实例化被测模块 led,并将其命名为 uut
.clk(clk), // 连接输入信号 clk
.reset(reset), // 连接输入信号 reset
.led(led) // 连接输出信号 led
);
always #10 clk =~clk; // 定义时钟信号 clk 为周期为20ns的反转信号
initial begin // 初始化模块
// Initialize Inputs
clk = 0; // 初始化时钟信号 clk 为 0
reset = 1; // 初始化复位信号 reset 为 1
// Wait 100 ns for global reset to finish
#100; // 等待100ns,使全局复位完成
reset = 0; // 将复位信号 reset 置为 0
// Add stimulus here // 在此处添加刺激信号
end
endmodule // 结束模块定义
```
阅读全文
相关推荐
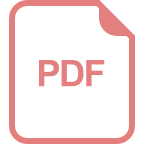








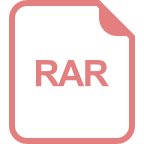
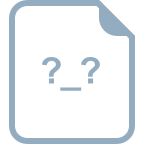
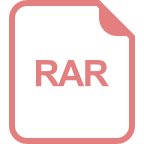
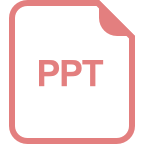