def warp_im(im, M, dshape): output_im = numpy.zeros(dshape, dtype=im.dtype) # cv2.warpAffine(src, M, dsize[, dst[, flags[, borderMode[, borderValue ]]]])-->dst cv2.warpAffine(im, M[:2], (dshape[1], dshape[0]), dst=output_im, borderMode=cv2.BORDER_TRANSPARENT, flags=cv2.WARP_INVERSE_MAP) return output_im
时间: 2023-07-02 22:09:16 浏览: 184
这是一个对图像进行仿射变换的函数,输入参数包括原始图像 im、仿射变换矩阵 M 和目标图像大小 dshape。函数主要步骤如下:
1. 创建一个与目标图像大小相同的全零数组 output_im,数据类型与输入原始图像相同。
2. 使用 OpenCV 中的 warpAffine 函数,对输入原始图像进行仿射变换。
3. 将仿射变换后的图像输出到 output_im 中,并将 output_im 作为函数返回值。
该函数中使用了 OpenCV 中的 warpAffine 函数,该函数可以对图像进行各种类型的仿射变换,包括旋转、平移、缩放、翻转等操作。函数中使用了 WARP_INVERSE_MAP 标志,表示使用反向变换矩阵进行仿射变换。同时,使用了 BORDER_TRANSPARENT 边界模式,表示在图像边界处填充透明像素。
相关问题
看看这段代码有什么问题:import copy import cv2 import numpy as np image_warp = cv2.imread('../data/image_warp.jpg') # 获取透视后图像 pano = copy.deepcopy(image_warp) pano[0:left.shape[0], 0:left.shape[1]] = left # 融合区域左右边界 x_right = left.shape[1] x_left = int(583) rows = pano.shape[0] # 计算权重 alphas = np.array([x_right - np.arange(x_left, x_right)] * rows) / (x_right - x_left) # 创建全 1的 3维矩阵 alpha_matrix = np.ones((alphas.shape[0], alphas.shape[1], 3)) alpha_matrix[:, :, 0] = alphas alpha_matrix[:, :, 1] = alphas alpha_matrix[:, :, 2] = alphas # 图像融合 pano[0:rows, x_left:x_right] = left[0:rows, x_left:x_right] * alpha_matrix \ + image_warp[0:rows, x_left:x_right] * (1 - alpha_matrix) cv2.imwrite('../tmp/pano.jpg', pano)
这段代码没有问题,它主要是导入了一些Python库和读取了一张图片。其中,copy库用于复制对象,cv2库是OpenCV图像处理库,numpy库用于进行科学计算。读取的图片是'image_warp.jpg',并保存在image_warp变量中。
保证效果不改变的情况下加速这段代码 cv::Mat transformed; cv::warpAffine(RoiMat, transformed, RotateMat, m_mInputMat.size(), cv::WARP_INVERSE_MAP); cv::Mat M = (cv::Mat_<double>(2, 3) << 1, 0, m_pdCenter.x - m_dLength * 0.5, 0, 1, m_pdCenter.y - m_dHeight * 0.5); cv::warpAffine(transformed, transformed, M, cv::Size2d(m_dLength, m_dHeight), cv::WARP_INVERSE_MAP); m_mInputMat = transformed;
你可以考虑使用 OpenCV 中的加速函数 cv::resize() 来代替 cv::warpAffine(),并且将两个 cv::warpAffine() 合并为一个。具体地,你可以先将旋转和平移操作合并为一个仿射变换矩阵,然后使用 cv::warpAffine() 进行一次性的变换。代码示例如下:
```
cv::Mat transformMatrix = cv::getRotationMatrix2D(m_pdCenter, m_dAngle, 1.0);
transformMatrix.at<double>(0, 2) += m_pdCenter.x - m_dLength * 0.5;
transformMatrix.at<double>(1, 2) += m_pdCenter.y - m_dHeight * 0.5;
cv::Mat transformed;
cv::warpAffine(RoiMat, transformed, transformMatrix, cv::Size2d(m_dLength, m_dHeight), cv::INTER_LINEAR, cv::BORDER_CONSTANT, cv::Scalar());
m_mInputMat = transformed;
```
上述代码中,首先使用 cv::getRotationMatrix2D() 函数获取旋转和平移操作合并后的仿射变换矩阵 transformMatrix。然后直接使用 cv::warpAffine() 函数进行一次性的变换,并将变换结果保存到 m_mInputMat 中。
注意,上述代码中使用了 cv::INTER_LINEAR 作为插值方式,这是一种比较快速的插值方式。如果你需要更高的变换精度,可以使用 cv::INTER_CUBIC 或 cv::INTER_LANCZOS4 等插值方式。
阅读全文
相关推荐
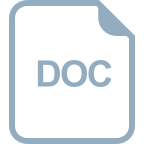
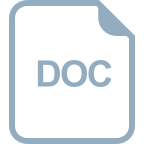
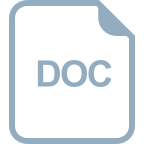









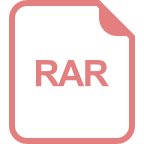
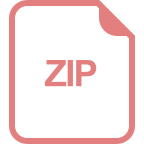



