为这段代码加上注释 def get_split_merge(img): h,w,c = img.shape img_upper = img[0:int(5/12*h),:] img_lower = img[int(1/3*h):,:] img_upper = cv2.resize(img_upper,(img_lower.shape[1],img_lower.shape[0])) new_img = np.hstack((img_upper,img_lower)) return new_img
时间: 2023-10-24 19:04:35 浏览: 38
好的,以下是代码注释:
```
def get_split_merge(img):
# 获取输入图像的高、宽和通道数
h, w, c = img.shape
# 将输入图像分为上下两部分,上部分高度为原图高度的 5/12,下部分高度为原图高度的 2/3
img_upper = img[0:int(5/12*h),:]
img_lower = img[int(1/3*h):,:]
# 将上部分图像缩放到与下部分图像相同的大小
img_upper = cv2.resize(img_upper, (img_lower.shape[1], img_lower.shape[0]))
# 将缩放后的上下两部分图像水平拼接
new_img = np.hstack((img_upper, img_lower))
# 返回拼接后的新图像
return new_img
```
这段代码的功能是将输入的图像分为上下两部分,将上部分缩放到与下部分相同的大小,然后将缩放后的上下两部分水平拼接,最终返回拼接后的新图像。
相关问题
给下列代码加注释: def merge_accumulate_client_update(self, list_num_proc, list_state_dict, lr): total_num_proc = sum(list_num_proc) # merged_state_dict = dict() dict_keys = list_state_dict[0].keys() for state_dict in list_state_dict[1:]: assert state_dict.keys() == dict_keys # accumulate extra sgrad and remove from state_dict if self.use_adaptive and self.is_adj_round(): prefix = "extra." for state_dict in list_state_dict: del_list = [] for key, param in state_dict.items(): if key[:len(prefix)] == prefix: sgrad_key = key[len(prefix):] mask_0 = self.model.get_mask_by_name(sgrad_key) == 0. dense_sgrad = torch.zeros_like(mask_0, dtype=torch.float) dense_sgrad.masked_scatter_(mask_0, param) # no need to divide by lr self.control.accumulate(sgrad_key, dense_sgrad) del_list.append(key) for del_key in del_list: del state_dict[del_key]
```python
def merge_accumulate_client_update(self, list_num_proc, list_state_dict, lr):
total_num_proc = sum(list_num_proc)
# merged_state_dict = dict()
dict_keys = list_state_dict[0].keys()
# Check if all state dicts have the same keys
for state_dict in list_state_dict[1:]:
assert state_dict.keys() == dict_keys
# accumulate extra sgrad and remove from state_dict
if self.use_adaptive and self.is_adj_round():
prefix = "extra."
for state_dict in list_state_dict:
del_list = []
for key, param in state_dict.items():
# Check if the key starts with 'extra.'
if key[:len(prefix)] == prefix:
# Get the corresponding sgrad key
sgrad_key = key[len(prefix):]
# Create a mask of zeroes
mask_0 = self.model.get_mask_by_name(sgrad_key) == 0.
# Create a dense tensor and fill it with values from param based on the mask
dense_sgrad = torch.zeros_like(mask_0, dtype=torch.float)
dense_sgrad.masked_scatter_(mask_0, param)
# Accumulate the dense sgrad without dividing by lr
self.control.accumulate(sgrad_key, dense_sgrad)
# Add the key to the delete list
del_list.append(key)
# Remove the keys from the state_dict
for del_key in del_list:
del state_dict[del_key]
```
这段代码实现了一个`merge_accumulate_client_update`方法,主要功能是合并和累加`list_state_dict`中的状态字典。以下是对代码的注释:
- `total_num_proc`:所有进程数的总和。
- `dict_keys`:状态字典的键列表。
- 检查所有状态字典是否具有相同的键。
- 如果使用自适应且处于调整轮次,则累加额外的`sgrad`并从状态字典中删除。
- `prefix`:额外`sgrad`的前缀。
- 对于每个状态字典,遍历键和参数。
- 如果键以`prefix`开头,则获取相应的`sgrad`键。
- 创建一个零填充的掩码。
- 创建一个稠密张量,并根据掩码从参数中填充值。
- 累加不除以`lr`的稠密`sgrad`。
- 将键添加到删除列表。
- 从状态字典中删除键。
img_merged = Image.merge("RGB", (r, g, b))
这行代码是将三个颜色通道(r、g、b)合并成一张 RGB 图像。Image.merge() 方法需要两个参数,第一个参数是图像模式("RGB" 表示三个颜色通道),第二个参数是一个元组,包含了每个通道的图像数据。在这个例子中,r、g、b 分别是三个颜色通道的图像数据,它们被合并成一张完整的 RGB 图像。
相关推荐
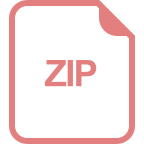
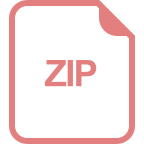













