用c++实现:设计一个字符串类重载“+=”运算符,使其实现两个字符串的连接并赋值。试分别用成员函数和友元函数实现。
时间: 2023-11-27 20:55:11 浏览: 123
首先,我们定义一个字符串类:
```cpp
#include <cstring>
class MyString {
public:
MyString(const char* str = nullptr);
MyString(const MyString& other);
~MyString();
MyString& operator=(const MyString& other);
const char* c_str() const;
private:
char* m_data;
};
MyString::MyString(const char* str) {
if (str == nullptr) {
m_data = new char[1];
*m_data = '\0';
} else {
m_data = new char[strlen(str) + 1];
strcpy(m_data, str);
}
}
MyString::MyString(const MyString& other) {
m_data = new char[strlen(other.m_data) + 1];
strcpy(m_data, other.m_data);
}
MyString::~MyString() {
delete[] m_data;
}
MyString& MyString::operator=(const MyString& other) {
if (this != &other) {
delete[] m_data;
m_data = new char[strlen(other.m_data) + 1];
strcpy(m_data, other.m_data);
}
return *this;
}
const char* MyString::c_str() const {
return m_data;
}
```
接下来,我们可以用成员函数和友元函数分别实现重载“+=”运算符:
```cpp
// 成员函数实现
MyString& MyString::operator+=(const MyString& other) {
char* temp = new char[strlen(m_data) + strlen(other.m_data) + 1];
strcpy(temp, m_data);
strcat(temp, other.m_data);
delete[] m_data;
m_data = temp;
return *this;
}
// 友元函数实现
MyString operator+(const MyString& str1, const MyString& str2) {
MyString temp;
delete[] temp.m_data;
temp.m_data = new char[strlen(str1.m_data) + strlen(str2.m_data) + 1];
strcpy(temp.m_data, str1.m_data);
strcat(temp.m_data, str2.m_data);
return temp;
}
MyString& MyString::operator+=(const MyString& other) {
*this = *this + other;
return *this;
}
```
使用示例:
```cpp
#include <iostream>
using namespace std;
int main() {
MyString str1("Hello");
MyString str2(" World!");
str1 += str2; // 成员函数实现
cout << str1.c_str() << endl;
MyString str3("Hello");
MyString str4(" C++!");
MyString str5 = str3 + str4; // 友元函数实现
cout << str5.c_str() << endl;
return 0;
}
```
阅读全文
相关推荐
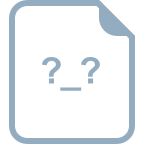







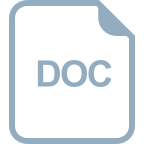
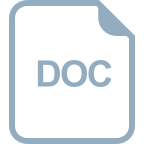
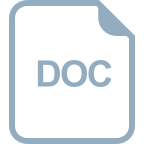




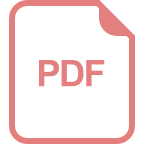