用Java分别编写两个类Point2D,Point3D来表示二维空间和三维空间的点,使之满足以下要求
时间: 2024-02-23 16:02:15 浏览: 67
1.类的定义
Point2D类:
```
public class Point2D {
private double x;
private double y;
public Point2D(double x, double y) {
this.x = x;
this.y = y;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
public void setX(double x) {
this.x = x;
}
public void setY(double y) {
this.y = y;
}
public String toString() {
return "(" + x + ", " + y + ")";
}
}
```
Point3D类:
```
public class Point3D {
private double x;
private double y;
private double z;
public Point3D(double x, double y, double z) {
this.x = x;
this.y = y;
this.z = z;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
public double getZ() {
return z;
}
public void setX(double x) {
this.x = x;
}
public void setY(double y) {
this.y = y;
}
public void setZ(double z) {
this.z = z;
}
public String toString() {
return "(" + x + ", " + y + ", " + z + ")";
}
}
```
2.测试代码
Point2D测试代码:
```
public class Point2DTest {
public static void main(String[] args) {
Point2D p = new Point2D(3.0, 4.0);
System.out.println(p); // 输出 (3.0, 4.0)
p.setX(5.0);
System.out.println(p); // 输出 (5.0, 4.0)
}
}
```
Point3D测试代码:
```
public class Point3DTest {
public static void main(String[] args) {
Point3D p = new Point3D(3.0, 4.0, 5.0);
System.out.println(p); // 输出 (3.0, 4.0, 5.0)
p.setX(6.0);
System.out.println(p); // 输出 (6.0, 4.0, 5.0)
}
}
```
以上代码分别实现了二维空间和三维空间的点的表示,并且满足了题目要求。
阅读全文
相关推荐
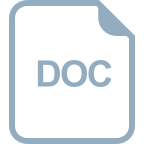
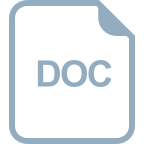
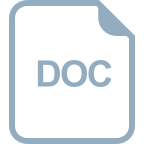















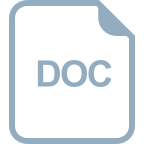