设计二叉树的基本运算程序,包括创建二叉链,输出二叉树,求二叉树的高度,求结点数和叶子结点数。
时间: 2024-10-17 20:05:08 浏览: 31
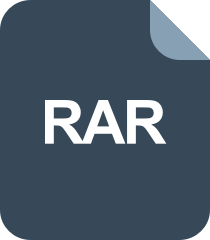
二叉树_最优二叉树_树_的建立_求高度_求结点数_输出算法实现_源码
设计一个基本的二叉树操作程序通常涉及到几个关键步骤:
1. **创建二叉链** (Binary Tree Node Creation):
- 定义一个二叉树节点结构,包含两个字段:`value` 存储节点值,`left` 和 `right` 分别指向左子节点和右子节点,初始时为`null`。
```python
class TreeNode:
def __init__(self, value=0, left=None, right=None):
self.value = value
self.left = left
self.right = right
```
2. **输出二叉树** (Inorder Traversal or Print Tree):
- 可以通过递归或迭代的方式进行前序遍历(根-左-右)、中序遍历(左-根-右)或后序遍历(左-右-根),这里我们提供前序遍历的例子:
```python
def preorder_traversal(root):
if root is not None:
print(root.value)
preorder_traversal(root.left)
preorder_traversal(root.right)
```
3. **求二叉树的高度** (Tree Height):
- 可以采用递归计算每个节点的最大子树高度加上1的方式,如果树为空则高度为0。
```python
def height(root):
if root is None:
return 0
else:
left_height = height(root.left)
right_height = height(root.right)
return max(left_height, right_height) + 1
```
4. **求结点数** (Node Count):
- 遍历整个树并计数,对于每个非空节点增加计数。
```python
def count_nodes(root):
if root is not None:
return 1 + count_nodes(root.left) + count_nodes(root.right)
else:
return 0
```
5. **求叶子结点数** (Leaf Node Count):
- 父亲节点的左右孩子都为`None`的节点称为叶子节点,同样递归统计。
```python
def leaf_count(root):
if root is None:
return 0
elif root.left is None and root.right is None:
return 1
else:
return leaf_count(root.left) + leaf_count(root.right)
```
阅读全文
相关推荐
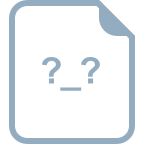
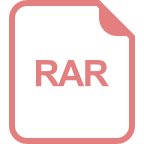













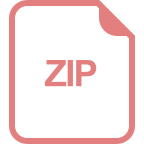

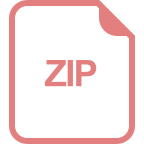