c语言利用两个栈s1和s2来模拟一个队列。用栈的运算来实现该队列的三个运算:inqueue——插入一个元素入队列;outqueue——删除一个元素出队列;queue_empty——判断队列为空
时间: 2023-11-10 12:05:26 浏览: 84
以下是利用两个栈s1和s2来模拟一个队列的C语言代码:
```
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 100
typedef struct {
int data[MAXSIZE];
int top;
} Stack;
void initStack(Stack *s) { // 初始化栈
s->top = -1;
}
void push(Stack *s, int x) { // 入栈操作
if (s->top == MAXSIZE-1) {
printf("Stack Overflow!\n");
return;
}
s->top ++;
s->data[s->top] = x;
}
int pop(Stack *s) { // 出栈操作
if (s->top == -1) {
printf("Stack Underflow!\n");
return -1;
}
int x = s->data[s->top];
s->top --;
return x;
}
int getTop(Stack *s) { // 获取栈顶元素
if (s->top == -1) {
printf("Stack Underflow!\n");
return -1;
}
int x = s->data[s->top];
return x;
}
int isEmpty(Stack *s) { // 判断栈是否为空
return s->top == -1;
}
typedef struct {
Stack s1;
Stack s2;
} Queue;
void initQueue(Queue *q) { // 初始化队列
initStack(&q->s1);
initStack(&q->s2);
}
void inqueue(Queue *q, int x) { // 入队操作
push(&q->s1, x);
}
int outqueue(Queue *q) { // 出队操作
if (isEmpty(&q->s1) && isEmpty(&q->s2)) {
printf("Queue Underflow!\n");
return -1;
}
if (isEmpty(&q->s2)) { // 如果s2为空,则将s1中的元素全部倒入s2中
while (!isEmpty(&q->s1)) {
int x = pop(&q->s1);
push(&q->s2, x);
}
}
int x = pop(&q->s2); // 此时s2的栈顶元素即为队列头部元素
return x;
}
int queue_empty(Queue *q) { // 判断队列是否为空
return isEmpty(&q->s1) && isEmpty(&q->s2);
}
int main() {
Queue q;
initQueue(&q);
inqueue(&q, 1);
inqueue(&q, 2);
inqueue(&q, 3);
printf("%d\n", outqueue(&q)); // 输出队列头部元素1
inqueue(&q, 4);
printf("%d\n", outqueue(&q)); // 输出队列头部元素2
printf("%d\n", outqueue(&q)); // 输出队列头部元素3
printf("%d\n", outqueue(&q)); // 输出队列头部元素4
printf("%d\n", outqueue(&q)); // 队列为空,输出-1(队列下溢)
return 0;
}
```
相关推荐
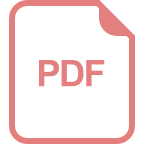
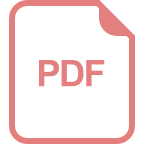
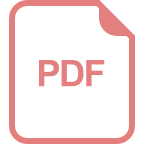














