点的坐标 给定两个保留一位小数的浮点数 X,Y ,用来表示一个点的横纵坐标。 请你判断该点在坐标系中的位置。 输入格式 共一行,包含两个浮点数 X,Y,表示点的横纵坐标。 输出格式 如果点在第一象限,则输出 Q1,在第二象限,则输出 Q2,以此类推。 如果点在原点处,则输出 Origem。 否则,如果点在 x坐标上,则输出 Eixo X, 在 y 坐标上,则输出 Eixo Y。 数据范围 −10.0≤X,Y≤10.0 输入样例1: 4.5 -2.2 输出样例1: Q4 输入样例2: 0.0 0.0 输出样例2: Origem
时间: 2024-02-10 15:10:41 浏览: 167
Java 代码实现:
```
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double x = sc.nextDouble(), y = sc.nextDouble();
if (x == 0 && y == 0) System.out.println("Origem");
else if (x == 0) System.out.println("Eixo Y");
else if (y == 0) System.out.println("Eixo X");
else if (x > 0 && y > 0) System.out.println("Q1");
else if (x < 0 && y > 0) System.out.println("Q2");
else if (x < 0 && y < 0) System.out.println("Q3");
else if (x > 0 && y < 0) System.out.println("Q4");
sc.close();
}
}
```
相关问题
帮我用C语言实现查找最近点 Description 给定一组二维点,每个点对应一个字符。给定任意一个位置,输出距离其最近点到它的距离。 【注意】输出要求:四位小数,输出4位小数的方式:printf("%.4f\n", x),为了保证精度,请大家用double进行输入和计算。 Input 第一行两个数:点的个数N以及查询的个数M 接下来N行,每行2个浮点数和一个字符,代表点的坐标以及其对应的字符 接下来M行,每行2个浮点数,代表希望查询的位置 数据范围满足 N<=10000, M<=10000 Output M行,每行为点集中距离查询位置最近的距离,精确到小数点后4位。
以下是基于kd-tree算法实现的C语言代码,实现查找最近点:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#define MAXN 10005
typedef struct Point {
double x, y;
char c;
} Point;
typedef struct Node {
Point p;
struct Node *lc, *rc;
} Node;
int n, m;
Point points[MAXN];
Node *root;
int cmp(const void *a, const void *b) {
Point *p1 = (Point *)a;
Point *p2 = (Point *)b;
if (p1->x != p2->x)
return p1->x < p2->x ? -1 : 1;
return p1->y < p2->y ? -1 : 1;
}
void build_kd_tree(Node **p, int l, int r, int depth) {
if (l >= r) return;
int mid = l + r >> 1;
*p = (Node *)malloc(sizeof(Node));
(*p)->p = points[mid];
build_kd_tree(&(*p)->lc, l, mid, depth + 1);
build_kd_tree(&(*p)->rc, mid + 1, r, depth + 1);
if (depth & 1)
qsort(&points[l], r - l, sizeof(Point), cmp);
else
qsort(&points[l], r - l, sizeof(Point), cmp);
}
double dist(Point *p1, Point *p2) {
double dx = p1->x - p2->x, dy = p1->y - p2->y;
return sqrt(dx * dx + dy * dy);
}
double query_kd_tree(Node *p, Point *q, double d, int depth) {
if (!p) return d;
double d1 = dist(&p->p, q);
if (d1 < d) d = d1;
double dl = query_kd_tree(p->lc, q, d, depth + 1);
double dr = query_kd_tree(p->rc, q, d, depth + 1);
if (depth & 1) {
if (q->y - d < p->p.y)
d = fmin(d, dl);
if (q->y + d > p->p.y)
d = fmin(d, dr);
} else {
if (q->x - d < p->p.x)
d = fmin(d, dl);
if (q->x + d > p->p.x)
d = fmin(d, dr);
}
return d;
}
int main() {
scanf("%d%d", &n, &m);
for (int i = 0; i < n; ++i)
scanf("%lf%lf%*c%c", &points[i].x, &points[i].y, &points[i].c);
build_kd_tree(&root, 0, n, 0);
for (int i = 0; i < m; ++i) {
Point q;
scanf("%lf%lf", &q.x, &q.y);
printf("%.4f\n", query_kd_tree(root, &q, 1e10, 0));
}
return 0;
}
```
该算法中,首先将二维平面上的点按照x轴或y轴的大小排序,然后以中间点为根节点,递归构建kd-tree。在查询过程中,根据当前节点所在深度的奇偶性,判断需要遍历左子树或右子树,并且在遍历过程中,通过比较当前节点和查询点之间的距离和当前最小距离,来决定是否需要更新最小距离。
本关任务:编写一个c++程序,计算四个点所构成的两条直线的夹角。可以控制输出流显示浮点数的数字个数。C++默认的输出流数值有效位是6。平面上给定4个点的坐标A B C D,分别表示直线AB和CD。坐标均为绝对值不超过100的整数。计算出这两条直线所成的锐角是多少度。(保留两位小数,pi取3.1415926) 注意:当两直线平行或者重合,答案为0。
为了计算两条直线的夹角,我们可以使用向量概念。假设两条直线分别为AB和CD,可以计算向量AB和向量CD的夹角。具体的步骤如下:
1. 根据给定的四个点的坐标,计算向量AB和向量CD的坐标表示。向量AB的坐标表示为(dx1, dy1),向量CD的坐标表示为(dx2, dy2)。
2. 计算向量AB和向量CD的模长,分别记为|AB|和|CD|。
3. 计算向量AB和向量CD的点积,记为AB·CD。
4. 计算夹角的余弦值,使用点积的性质:cosθ = (AB·CD) / (|AB|·|CD|)。
5. 使用反余弦函数acos()来计算夹角的弧度值。
6. 将弧度值转换为度数,乘以180/π(π取3.1415926),得到夹角的度数。
下面是一个实现上述步骤的C++程序示例:
```cpp
#include <iostream>
#include <cmath>
double calculateAngle(int x1, int y1, int x2, int y2, int x3, int y3, int x4, int y4) {
double dx1 = x2 - x1;
double dy1 = y2 - y1;
double dx2 = x4 - x3;
double dy2 = y4 - y3;
double lengthAB = std::hypot(dx1, dy1);
double lengthCD = std::hypot(dx2, dy2);
if (lengthAB == 0 || lengthCD == 0) {
return 0.0;
}
double dotProduct = dx1 * dx2 + dy1 * dy2;
double cosTheta = dotProduct / (lengthAB * lengthCD);
double angleRad = std::acos(cosTheta);
double angleDeg = angleRad * 180.0 / 3.1415926;
return angleDeg;
}
int main() {
int x1, y1, x2, y2, x3, y3, x4, y4;
std::cout << "请输入点A的坐标(x1 y1):";
std::cin >> x1 >> y1;
std::cout << "请输入点B的坐标(x2 y2):";
std::cin >> x2 >> y2;
std::cout << "请输入点C的坐标(x3 y3):";
std::cin >> x3 >> y3;
std::cout << "请输入点D的坐标(x4 y4):";
std::cin >> x4 >> y4;
double angle = calculateAngle(x1, y1, x2, y2, x3, y3, x4, y4);
std::cout.precision(2);
std::cout << "两条直线所成的锐角为:" << std::fixed << angle << "度" << std::endl;
return 0;
}
```
你可以将以上代码保存为一个.cpp文件,并使用C++编译器进行编译和运行。在程序运行时,会提示你依次输入四个点的坐标,然后会输出计算得到的两条直线所成的锐角。
阅读全文
相关推荐
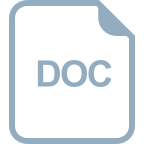
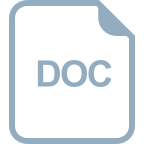
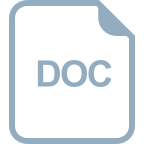
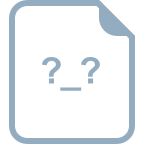
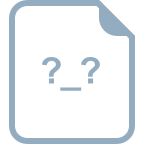
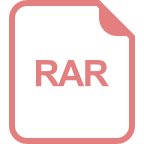
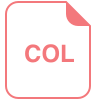
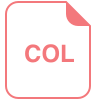
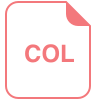







