unity webgl怎么通过StreamingAssets的方式点击不同按钮播放mp4格式视频的操作方式和完整代码,按钮和视频10个以上
时间: 2024-03-09 14:49:29 浏览: 19
首先,需要将MP4格式的视频文件放入Unity项目的StreamingAssets文件夹中。然后创建一个Canvas,在Canvas上添加若干个Button组件用于触发播放不同的视频。
接下来,创建一个名为“VideoPlayer”的C#脚本,并将其附加到任意一个GameObject上。在脚本中,我们需要使用Unity的VideoPlayer组件来播放视频,并且需要使用Button组件来监听按钮的点击事件。
以下是完整的代码示例:
```
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.Video;
public class VideoPlayer : MonoBehaviour
{
public List<string> videoFileNames; // 视频文件名列表
public RawImage image; // 用于显示视频的RawImage组件
public Button[] buttons; // 用于触发播放不同视频的按钮组件
private VideoPlayer player; // 视频播放器
private int currentVideoIndex; // 当前播放的视频索引
void Start()
{
player = gameObject.AddComponent<VideoPlayer>();
player.playOnAwake = false;
player.renderMode = VideoRenderMode.RenderTexture;
player.targetTexture = new RenderTexture(1920, 1080, 16, RenderTextureFormat.ARGB32);
image.texture = player.targetTexture;
for (int i = 0; i < buttons.Length; i++)
{
int index = i; // 保存当前按钮的索引
buttons[i].onClick.AddListener(delegate { PlayVideo(index); });
}
currentVideoIndex = -1; // 初始化当前视频索引
}
void PlayVideo(int index)
{
if (index < 0 || index >= videoFileNames.Count) return;
if (currentVideoIndex == index) // 当前正在播放该视频,不需要重新加载
{
player.Play();
return;
}
currentVideoIndex = index;
player.Stop();
string videoPath = Application.streamingAssetsPath + "/" + videoFileNames[index];
player.url = videoPath;
player.Prepare();
player.Play();
}
}
```
在代码中,需要注意的是:
1. videoFileNames是一个字符串列表,用于保存所有视频文件的文件名。在实际使用时,需要将所有视频文件的文件名添加到这个列表中。
2. image是一个RawImage组件,用于显示视频。
3. buttons是一个Button组件数组,用于触发播放不同视频的事件。在实际使用时,需要将所有的Button组件添加到这个数组中。
4. 在Start函数中,我们创建了一个VideoPlayer组件,并将其附加到当前GameObject上。然后,我们设置了VideoPlayer的一些属性,比如playOnAwake、renderMode和targetTexture。
5. 在PlayVideo函数中,我们首先判断index是否有效。然后,我们判断当前是否正在播放该视频,如果是,则不需要重新加载视频文件,直接播放即可。如果不是,则需要重新加载视频文件,并且将currentVideoIndex设置为index。
最后,将VideoPlayer脚本附加到任意一个GameObject上,并将所有需要播放的视频文件添加到StreamingAssets文件夹中即可。
相关推荐






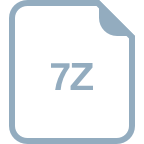
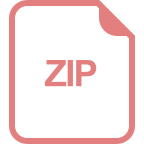
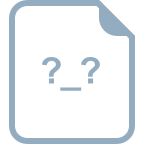
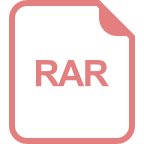
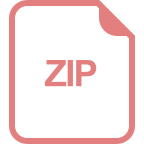
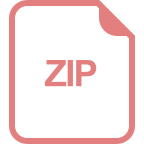
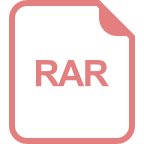