如何用opencv检测方形轮廓并标记轮廓上的缺口
时间: 2024-05-13 09:16:21 浏览: 27
要检测方形轮廓并标记轮廓上的缺口,可以按照以下步骤操作:
1. 导入OpenCV库并读入图像。
```python
import cv2
img = cv2.imread('image.jpg')
```
2. 将图像转换为灰度图像并进行二值化处理。
```python
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
_, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
```
3. 查找轮廓并筛选出符合条件的轮廓(如面积、周长等)。
```python
contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
area = cv2.contourArea(contour)
perimeter = cv2.arcLength(contour, True)
if area > 1000 and perimeter > 100:
approx = cv2.approxPolyDP(contour, 0.01*perimeter, True)
if len(approx) == 4:
# 处理缺口
```
4. 对于符合条件的轮廓,使用 `cv2.approxPolyDP` 函数对其进行多边形逼近,得到近似的四边形轮廓。
```python
approx = cv2.approxPolyDP(contour, 0.01*perimeter, True)
if len(approx) == 4:
# 处理缺口
```
5. 对于四边形轮廓,使用 `cv2.drawContours` 函数绘制轮廓,并在轮廓上标记缺口。
```python
cv2.drawContours(img, [approx], -1, (0, 0, 255), 2)
for i in range(len(approx)):
p1 = tuple(approx[i][0])
p2 = tuple(approx[(i+1) % len(approx)][0])
distance = cv2.norm(p1-p2)
if distance < 20:
cv2.circle(img, p2, 5, (0, 255, 0), -1)
```
完整代码如下:
```python
import cv2
img = cv2.imread('image.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
_, thresh = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
contours, _ = cv2.findContours(thresh, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
area = cv2.contourArea(contour)
perimeter = cv2.arcLength(contour, True)
if area > 1000 and perimeter > 100:
approx = cv2.approxPolyDP(contour, 0.01*perimeter, True)
if len(approx) == 4:
cv2.drawContours(img, [approx], -1, (0, 0, 255), 2)
for i in range(len(approx)):
p1 = tuple(approx[i][0])
p2 = tuple(approx[(i+1) % len(approx)][0])
distance = cv2.norm(p1-p2)
if distance < 20:
cv2.circle(img, p2, 5, (0, 255, 0), -1)
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关推荐
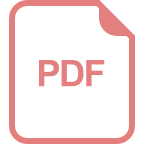
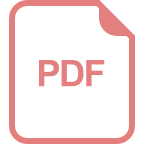
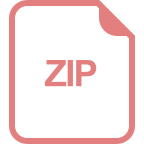
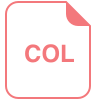
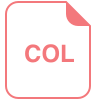
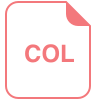
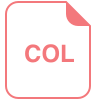
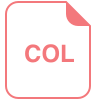









