QWidget中添加QGraphicsScene,QGraphicsScene中添加QWidget,QWidget又嵌入QLabel,如何获得QGraphicsScene鼠标点击下的坐标 C++
时间: 2024-03-04 14:48:16 浏览: 54
如果您想在C++中获取QGraphicsScene中鼠标事件的坐标,可以重载QGraphicsScene的`mousePressEvent()`函数,并将其作为事件过滤器安装到QGraphicsScene上。在`mousePressEvent()`函数中,您可以使用`QGraphicsSceneMouseEvent`参数中的`scenePos()`函数获取鼠标在场景中的坐标。接下来,您可以使用`QGraphicsView`的`mapFromScene()`函数将场景坐标转换为视图坐标,然后使用QWidget的`mapFromGlobal()`函数将视图坐标转换为QWidget坐标,最后使用QLabel的`mapFrom()`函数将QWidget坐标转换为QLabel坐标。下面是一个示例代码:
``` c++
#include <QGraphicsScene>
#include <QGraphicsView>
#include <QLabel>
#include <QWidget>
class MyWidget : public QWidget
{
Q_OBJECT
public:
MyWidget(QWidget *parent = nullptr) : QWidget(parent)
{
m_label = new QLabel("Hello World", this);
m_label->move(10, 10);
m_scene = new QGraphicsScene(this);
m_scene->addWidget(m_label);
QGraphicsView *view = new QGraphicsView(m_scene, this);
view->setGeometry(0, 0, 200, 200);
m_scene->installEventFilter(this);
}
protected:
bool eventFilter(QObject *obj, QEvent *event) override
{
if (obj == m_scene && event->type() == QEvent::GraphicsSceneMousePress)
{
QGraphicsSceneMouseEvent *mouse_event = static_cast<QGraphicsSceneMouseEvent*>(event);
QPointF pos = mouse_event->scenePos();
QPointF view_pos = m_scene->views()[0]->mapFromScene(pos);
QPoint widget_pos = mapToGlobal(view_pos.toPoint());
QPoint label_pos = m_label->mapFrom(this, widget_pos);
qDebug() << "Label position: " << label_pos;
}
return QWidget::eventFilter(obj, event);
}
private:
QGraphicsScene *m_scene;
QLabel *m_label;
};
```
在上面的代码中,我们在QWidget中创建了一个QLabel,并将其添加到QGraphicsScene中。然后,我们创建了一个QGraphicsView并将QGraphicsScene设置为其场景。接下来,我们安装了一个事件过滤器来捕获场景中的鼠标事件,并在事件过滤器中获取了鼠标坐标。最后,我们使用QWidget和QLabel的坐标转换函数来计算标签的坐标。
阅读全文
相关推荐
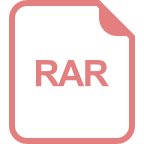
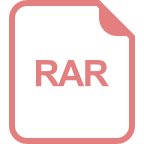
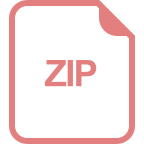
















