以高校为基本单位进行信息存放,高校信息包括:编号、学校名称、地址、省份、建校时间等。 功能要求: 1)能够对高校信息进行维护:新建、修改、删除操作: 2)按照高校任意信息进行查询: 3)以文件形式保存相关信息,可以读取默认文件中的信息进行查询等操作。c++
时间: 2023-11-28 22:49:05 浏览: 26
以下是一个简单的C++程序,实现了基本的高校信息维护和查询功能,包含以下几个类:
- School:高校类,包含编号、学校名称、地址、省份、建校时间等信息;
- SchoolDatabase:高校数据库类,用于维护和查询高校信息;
- FileHandler:文件处理类,用于保存和读取高校信息。
```
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
class School {
public:
int id;
string name;
string address;
string province;
string founded;
School(int id, string name, string address, string province, string founded) {
this->id = id;
this->name = name;
this->address = address;
this->province = province;
this->founded = founded;
}
void print() {
cout << "ID: " << id << endl
<< "Name: " << name << endl
<< "Address: " << address << endl
<< "Province: " << province << endl
<< "Founded: " << founded << endl;
}
};
class SchoolDatabase {
private:
vector<School> schools;
public:
void addSchool(School school) {
schools.push_back(school);
}
void removeSchool(int id) {
for (int i = 0; i < schools.size(); i++) {
if (schools[i].id == id) {
schools.erase(schools.begin() + i);
break;
}
}
}
void updateSchool(int id, School school) {
for (int i = 0; i < schools.size(); i++) {
if (schools[i].id == id) {
schools[i] = school;
break;
}
}
}
vector<School> searchSchool(string keyword) {
vector<School> result;
for (int i = 0; i < schools.size(); i++) {
if (schools[i].name.find(keyword) != string::npos ||
schools[i].address.find(keyword) != string::npos ||
schools[i].province.find(keyword) != string::npos ||
schools[i].founded.find(keyword) != string::npos) {
result.push_back(schools[i]);
}
}
return result;
}
};
class FileHandler {
public:
void saveToFile(string filename, SchoolDatabase db) {
ofstream outFile(filename);
if (outFile.is_open()) {
for (int i = 0; i < db.schools.size(); i++) {
outFile << db.schools[i].id << ","
<< db.schools[i].name << ","
<< db.schools[i].address << ","
<< db.schools[i].province << ","
<< db.schools[i].founded << endl;
}
outFile.close();
}
}
SchoolDatabase readFromFile(string filename) {
SchoolDatabase db;
ifstream inFile(filename);
if (inFile.is_open()) {
string line;
while (getline(inFile, line)) {
int pos1 = line.find(",");
int id = stoi(line.substr(0, pos1));
line = line.substr(pos1 + 1);
int pos2 = line.find(",");
string name = line.substr(0, pos2);
line = line.substr(pos2 + 1);
int pos3 = line.find(",");
string address = line.substr(0, pos3);
line = line.substr(pos3 + 1);
int pos4 = line.find(",");
string province = line.substr(0, pos4);
line = line.substr(pos4 + 1);
string founded = line;
School school(id, name, address, province, founded);
db.addSchool(school);
}
inFile.close();
}
return db;
}
};
int main() {
FileHandler fileHandler;
SchoolDatabase db = fileHandler.readFromFile("schools.txt");
while (true) {
cout << "-----------------------" << endl
<< "1. Add a school" << endl
<< "2. Remove a school" << endl
<< "3. Update a school" << endl
<< "4. Search schools" << endl
<< "5. Save to file" << endl
<< "6. Exit" << endl
<< "-----------------------" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1: {
int id;
string name, address, province, founded;
cout << "Enter ID: ";
cin >> id;
cout << "Enter name: ";
cin >> name;
cout << "Enter address: ";
cin >> address;
cout << "Enter province: ";
cin >> province;
cout << "Enter founded: ";
cin >> founded;
School school(id, name, address, province, founded);
db.addSchool(school);
break;
}
case 2: {
int id;
cout << "Enter ID: ";
cin >> id;
db.removeSchool(id);
break;
}
case 3: {
int id;
string name, address, province, founded;
cout << "Enter ID: ";
cin >> id;
cout << "Enter name: ";
cin >> name;
cout << "Enter address: ";
cin >> address;
cout << "Enter province: ";
cin >> province;
cout << "Enter founded: ";
cin >> founded;
School school(id, name, address, province, founded);
db.updateSchool(id, school);
break;
}
case 4: {
string keyword;
cout << "Enter keyword: ";
cin >> keyword;
vector<School> result = db.searchSchool(keyword);
for (int i = 0; i < result.size(); i++) {
result[i].print();
cout << endl;
}
break;
}
case 5: {
fileHandler.saveToFile("schools.txt", db);
break;
}
case 6: {
return 0;
}
default: {
cout << "Invalid choice" << endl;
break;
}
}
}
return 0;
}
```
注意:以上代码仅为示例,可能存在一些问题和不足,需要根据实际需求进行修改和完善。
相关推荐
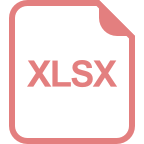
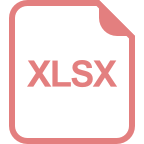
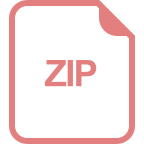














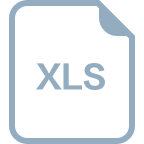