如何用函数实现一个pygame打地鼠游戏
时间: 2023-06-13 13:06:39 浏览: 45
下面是一个简单的pygame打地鼠游戏的函数实现:
```python
import pygame
import random
pygame.init()
# 设置窗口大小
WINDOW_WIDTH = 640
WINDOW_HEIGHT = 480
WINDOW_SIZE = (WINDOW_WIDTH, WINDOW_HEIGHT)
# 设置颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
GREEN = (0, 255, 0)
RED = (255, 0, 0)
# 设置字体
FONT = pygame.font.SysFont('arial', 24)
# 设置游戏参数
MOLE_SIZE = 50
MOLE_SPEED = 5
MOLE_SCORE = 10
GAME_TIME = 30
# 初始化游戏
def init_game():
# 创建窗口
window = pygame.display.set_mode(WINDOW_SIZE)
pygame.display.set_caption('打地鼠')
# 加载图片
mole_image = pygame.image.load('mole.png').convert_alpha()
# 创建地鼠
mole_rect = mole_image.get_rect()
mole_rect.centerx = random.randint(MOLE_SIZE, WINDOW_WIDTH - MOLE_SIZE)
mole_rect.centery = random.randint(MOLE_SIZE, WINDOW_HEIGHT - MOLE_SIZE)
# 初始化游戏状态
score = 0
time_left = GAME_TIME * 1000
game_over = False
return window, mole_image, mole_rect, score, time_left, game_over
# 绘制游戏界面
def draw_game(window, mole_image, mole_rect, score, time_left):
# 绘制背景
window.fill(WHITE)
# 绘制地鼠
window.blit(mole_image, mole_rect)
# 绘制得分和时间
score_text = FONT.render('得分:{}'.format(score), True, BLACK)
window.blit(score_text, (10, 10))
time_text = FONT.render('时间:{:.1f}'.format(time_left / 1000), True, BLACK)
window.blit(time_text, (WINDOW_WIDTH - time_text.get_width() - 10, 10))
# 刷新屏幕
pygame.display.flip()
# 处理游戏事件
def handle_events():
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 更新游戏状态
def update_game(mole_rect):
# 移动地鼠
mole_rect.move_ip(random.randint(-MOLE_SPEED, MOLE_SPEED), random.randint(-MOLE_SPEED, MOLE_SPEED))
# 碰撞检测
if mole_rect.left < 0 or mole_rect.right > WINDOW_WIDTH or mole_rect.top < 0 or mole_rect.bottom > WINDOW_HEIGHT:
mole_rect.centerx = random.randint(MOLE_SIZE, WINDOW_WIDTH - MOLE_SIZE)
mole_rect.centery = random.randint(MOLE_SIZE, WINDOW_HEIGHT - MOLE_SIZE)
# 更新游戏状态
return mole_rect
# 主函数
def main():
# 初始化游戏
window, mole_image, mole_rect, score, time_left, game_over = init_game()
# 游戏循环
clock = pygame.time.Clock()
while not game_over:
# 处理事件
handle_events()
# 更新游戏状态
mole_rect = update_game(mole_rect)
# 绘制游戏界面
draw_game(window, mole_image, mole_rect, score, time_left)
# 更新时间
time_left -= clock.tick(60)
# 判断游戏是否结束
if time_left < 0:
game_over = True
# 显示游戏结束界面
window.fill(WHITE)
score_text = FONT.render('得分:{}'.format(score), True, BLACK)
window.blit(score_text, (WINDOW_WIDTH // 2 - score_text.get_width() // 2, WINDOW_HEIGHT // 2 - score_text.get_height() // 2))
pygame.display.flip()
# 等待退出
while True:
handle_events()
if __name__ == '__main__':
main()
```
这个函数实现的pygame打地鼠游戏比较简单,只有一个地鼠,玩家需要在有限的时间内打中地鼠来得分。游戏结束后会显示得分。这个函数可以作为一个模板,你可以根据自己的需求进行修改和扩展。
相关推荐
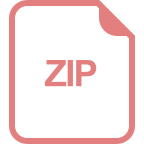












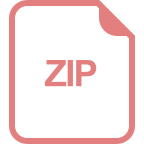
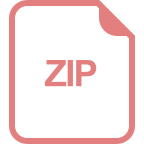