以tk主界面一个按钮指令作为pygame限时计分打地鼠游戏开始的打地鼠函数
时间: 2024-04-30 19:19:59 浏览: 10
好的,以下是一个简单的示例代码:
``` python
import pygame
import random
# 初始化 pygame
pygame.init()
# 设置游戏窗口大小
WINDOW_SIZE = (500, 500)
screen = pygame.display.set_mode(WINDOW_SIZE)
# 设置游戏标题
pygame.display.set_caption("打地鼠游戏")
# 加载地鼠图片
mouse_image = pygame.image.load("mouse.png")
# 设置游戏字体
font = pygame.font.SysFont(None, 48)
# 定义游戏时间(秒)
GAME_TIME = 30
# 定义计分板
score = 0
# 定义地鼠的出现位置
mouse_x = random.randint(50, 450)
mouse_y = random.randint(50, 450)
# 定义游戏结束的标志位
game_over = False
# 定义游戏开始时间
start_time = pygame.time.get_ticks()
# 定义打地鼠函数
def whack_a_mole():
global score, mouse_x, mouse_y, game_over, start_time
# 游戏循环
while not game_over:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.MOUSEBUTTONDOWN:
# 判断是否打中了地鼠
if mouse_rect.collidepoint(pygame.mouse.get_pos()):
score += 1
mouse_x = random.randint(50, 450)
mouse_y = random.randint(50, 450)
# 绘制游戏界面
screen.fill((255, 255, 255))
mouse_rect = screen.blit(mouse_image, (mouse_x, mouse_y))
score_text = font.render("分数:" + str(score), True, (0, 0, 0))
screen.blit(score_text, (20, 20))
# 判断游戏时间是否结束
elapsed_time = (pygame.time.get_ticks() - start_time) / 1000
if elapsed_time >= GAME_TIME:
game_over = True
end_text = font.render("游戏结束", True, (0, 0, 0))
screen.blit(end_text, (200, 200))
else:
time_text = font.render("时间:" + str(int(GAME_TIME - elapsed_time)), True, (0, 0, 0))
screen.blit(time_text, (350, 20))
# 更新屏幕
pygame.display.update()
# 主函数
def main():
# 等待用户点击按钮开始游戏
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
return
elif event.type == pygame.MOUSEBUTTONDOWN:
if event.button == 1:
# 开始游戏
whack_a_mole()
# 重置计分板
score = 0
# 重置游戏开始时间
start_time = pygame.time.get_ticks()
# 绘制游戏界面
screen.fill((255, 255, 255))
start_text = font.render("点击按钮开始游戏", True, (0, 0, 0))
screen.blit(start_text, (150, 200))
# 更新屏幕
pygame.display.update()
if __name__ == "__main__":
main()
```
这个程序分为两部分,第一部分是等待用户点击按钮开始游戏,第二部分是打地鼠游戏。在第一部分中,我们等待用户点击鼠标左键,然后进入打地鼠游戏。在第二部分中,我们定义了一个 `whack_a_mole()` 函数来处理打地鼠游戏的逻辑。游戏开始时,我们随机生成地鼠的出现位置,然后进入游戏循环,等待用户点击地鼠。如果用户点击了地鼠,那么我们就更新分数并随机生成下一个地鼠的位置。游戏结束时,我们显示游戏结束的文本,并等待用户再次点击鼠标左键重新开始游戏。
相关推荐
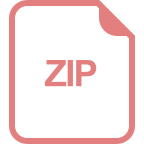













