java代码实现android自动连接ble设备
时间: 2023-06-05 09:04:28 浏览: 395
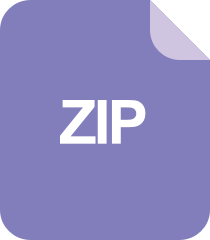
blessed-bluez:使用Java和Bluez的BLE库
你好,关于Java代码实现Android自动连接BLE设备的问题,我可以为您提供一些基本的思路和参考代码。首先,您需要使用Android中的BLE API来搜索和连接BLE设备。然后,您可以使用服务和特征值来与设备进行通信。以下是一些可能有用的示例代码:
1. 初始化BLE适配器和回调函数
```
BluetoothManager bluetoothManager = (BluetoothManager) getSystemService(Context.BLUETOOTH_SERVICE);
BluetoothAdapter mBluetoothAdapter = bluetoothManager.getAdapter();
BluetoothGatt mBluetoothGatt;
//设备连接状态回调函数
private final BluetoothGattCallback mGattCallback = new BluetoothGattCallback() {
@Override
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) {
if (newState == BluetoothProfile.STATE_CONNECTED) {
Log.d(TAG, "Connected to GATT server.");
mBluetoothGatt.discoverServices(); //连接成功之后搜索设备的服务
} else if (newState == BluetoothProfile.STATE_DISCONNECTED) {
Log.d(TAG, "Disconnected from GATT server.");
}
}
};
```
2. 搜索BLE设备
```
//BLE设备广播回调函数
private final BroadcastReceiver mGattUpdateReceiver = new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
final String action = intent.getAction();
if (BluetoothDevice.ACTION_FOUND.equals(action)) {
//获取扫描到的设备信息
BluetoothDevice device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE);
if (device.getName() != null) {
Log.d(TAG, "Found device: " + device.getName());
if (device.getName().equals("My BLE Device")) { //如果找到指定设备
//停止扫描并连接设备
mBluetoothAdapter.stopLeScan(mLeScanCallback);
mBluetoothGatt = device.connectGatt(getApplicationContext(), false, mGattCallback);
}
}
}
}
};
//开始搜索设备
private void scanBLEDevices() {
mHandler.postDelayed(new Runnable() {
@Override
public void run() {
mBluetoothAdapter.stopLeScan(mLeScanCallback);
}
}, 10000); //设置扫描时间为10s
mBluetoothAdapter.startLeScan(mLeScanCallback);
}
//设备搜索回调函数
private BluetoothAdapter.LeScanCallback mLeScanCallback = new BluetoothAdapter.LeScanCallback() {
@Override
public void onLeScan(final BluetoothDevice device, int rssi, byte[] scanRecord) {
runOnUiThread(new Runnable() {
@Override
public void run() {
Log.d(TAG, "Found device: " + device.getName());
}
});
}
};
```
3. 连接BLE设备并搜索服务
```
//连接设备
private void connectToDevice(String deviceAddress) {
BluetoothDevice device = mBluetoothAdapter.getRemoteDevice(deviceAddress);
mBluetoothGatt = device.connectGatt(this, false, mGattCallback);
}
//搜索服务
private void discoverServices() {
if (mBluetoothGatt != null) {
mBluetoothGatt.discoverServices();
}
}
//服务搜索回调函数
@Override
public void onServicesDiscovered(BluetoothGatt gatt, int status) {
if (status == BluetoothGatt.GATT_SUCCESS) {
List<BluetoothGattService> services = gatt.getServices();
for (BluetoothGattService service : services) {
Log.d(TAG, "Found service: " + service.getUuid());
}
} else {
Log.d(TAG, "Service discovery failed.");
}
}
```
以上是简单的示例代码,您可以根据自己的需求进行相应的更改和扩展。同时,也需要注意安全与隐私问题,确保BLE设备的安全连接和数据传输。希望能够对您有所帮助。
阅读全文
相关推荐
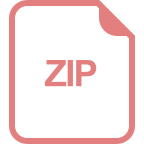
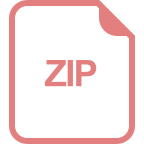
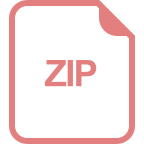
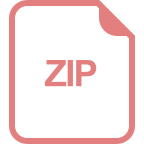
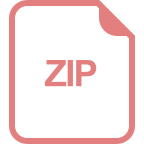
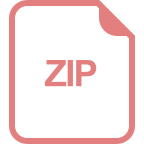
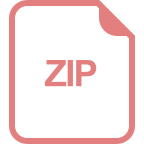
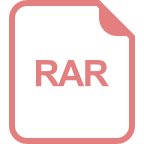
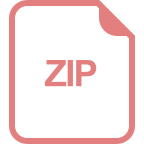
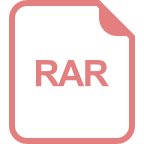
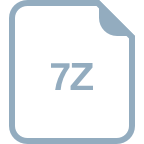
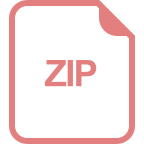
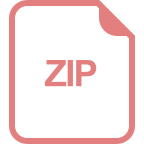
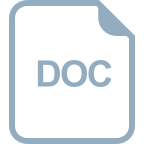
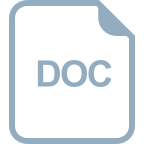
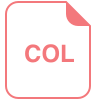